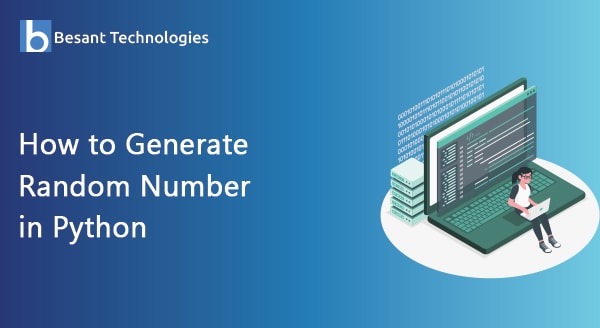
How to Generate Random Number in Python
Introduction
Programs need to create different items in case of building software. The case scenario can be seen in various applications like gambling, OTP generation, gaming, and more. With Python built-in functions, you can make the process of values generation easier and effective. Today in this tutorial, we will learn in-depth about generating a random number using various Python built-in functions.
What do you mean by Random Generation in Python?
Generators are described as functions that create items whenever they are being called. Generating random number sin Python is the built-in function which enables you to make number whenever needed. The built-in features can be embedded within Python’s random modules.
Below are some of the built-in functions of random number generation explained with their features in the random module.
- randrange() – capable of returning random values in between the particular interval and limit.
- choice() – ability to return a sequential random number.
- sample() – capable of returning an item that is selected randomly from any sequence.
- seed() – The values produced by these functions are meaningful and deterministic. The same values in the sequence are generated when there is the same seed number.
- randint() – Ability to return an integer selected randomly between the provided limit.
- shuffle() – helps to shuffles the provided sequence.
- uniform() – ability to return the values of floating-point between the provided range.
How to generate integers?
Random integers are generated with the help of built-in functions like randint() and randrange().
randint()
The built-in functions help to generate the integers between the provided limit. It makes use of the two critical parameters. The first parameters indicated the lower limit, whereas the second parameter indicates the upper limit. Let’s say, radiant(x,y) generates values from x to y, namely.
x<=a<=y (includes x and y)
Let’s take an example.
Import random random.randint(4,8)
Output:
6
The code above is used to generate any number from 4 to 8, but it should be within the provided limit. If you need to create many values between those ranges, you can use the for a loop. The code will look like.
Example:
Import random For a in range(4): print(random.randint(4,8))
Output:
4 5 6 8
You can make use of the randrange() function if you need to generate numbers in intervales. Let’s have a look at it below.
randrange():
The built-in function randrange() enables the users to produce values at regular intervals. Let’s check out an example.
Import random For a in range(6): print(random.randrange(4,40,4))
Output:
22 14 28 10 28 32
Have a look at the output, and you can see every number produced is the even number. Apart from this, you can make use of the random module built-in functions to generate floating-point values.
How to generate floating-point values?
You can use the built-in function uniform() and random() to generate floating-point numbers.
random()
The built-in function of this type generates floating-point values between 0.0 and 1.0. Therefore it does not include any parameters. The maximum value of this is 9.999, as the upper limit is excluded in this function.
Let’s check out an example.
Import random For a in range(4) print(random.random())
Output:
0.12787535455 0.194758765038 0.557557039302 0.03274744477458
uniform()
The built-in function uniform() is completely different from random() function. Here it makes use of two parameters, which indicates the lower and upper limits, respectively.
Let’s check out an example.
For a in range(4): print(random.uniform(5))
Output:
2.847374385985 4.7374734874 3.7374374934 4.77347374904
Python also helps you in generating random numbers from a provided sequence. Let’s check them below.
How to generate a random number from a provided sequence?
The built-in functions sample() and choice() are used for generating random numbers using a provided sequence in Python.
choice()
The built-in functions of this type take one sequence as a parameter and then returns the random number from the value.
Let’s take an example.
For a in range(4): Print(random.choice([1,2,3,4,7,8,9]))
Output:
2 1 7 3
From the above example, you can check out that the four values are returned by using the for loop, and all of the random numbers are taken from the provided list.
sample()
The built-in function sample() help you to choose some of the sequences randomly from the provided sequence and then results as output. It makes use of the two parameters, in which the first parameters indicate a sequence, and the second is an integer number specifying how many values should be returned in the result.
Let’s take an example.
print(random.sample([1,3,5,2,6,8,9],7))
Output:
[1,7,6,9]
The above result indicates that the output holds four random numbers selected from the provided sequence.
How to generate random values using shuffle()
The built-in function shuffle() is used for shuffling the provided sequence randomly.
Let’s take an example.
mylist=[1,3,5,7,9,2,4,6,8] random.shuffle(mylist) print(mylist)
Output:
[3,9,2,5,4,1,6,8,7]
How to generate random values using seed()
The built-in function seed() makes use of the number as a parameter known as the seed and generates the same random value every time whenever the function is called with that number.
Let’s check this with a clear example.
random.seed(1) print(random.random(), random.random(), random.random(), end=”nn”) Random. seed(2) print(random.random(), random.random(), random.random(), end=”nn”) random.seed(1) print(random.random(), random.random(), random.random(), end=”nn”) random.seed(2) print(random.random(), random.random(), random.random())
Output
0.374374327403740734 0.5473438057475057075 0.03276463446 0.448758745987459578 0.8264836478364764745 0.623463647348 0.374374327403740734 0.5473438057475057075 0.03276463446 0.448758745987459578 0.8264836478364764745 0.623463647348
In the above output, you can see the result for seed(1), and seed(2) is the same every time it’s called. The built-in functions are most important in experiments where you have to make use of the same random values to different test cases. Any other queries related to generating random numbers in Python? Let us know through the comment section below. To know more about Python and its applications, contact us!
Related Blogs:
- Brief Overview of Python Language
- Python Career opportunities
- Python Break Continue
- Python Control Flow
- Python Data Types
- Python Dictionary
- Python Exception Handling
- Python File
- Python Functions
- Python Substring
- Hash Table and Hash Map in Python