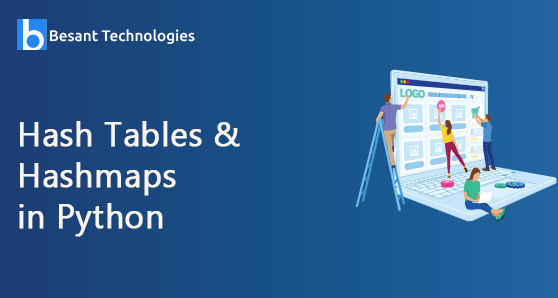
Hash Tables and Hashmaps in Python
Hash Tables and Hashmaps in Python
Data needs different ways to be accessed and stored. One significant implementation of data is the Hash Tables. When it comes to Python, Hash tables are used via dictionary ie, the built-in data type. Today in our blog, we can check out the in-depth tutorial on the hash table and hashmaps in Python, and how you can make use of them with the help of built-in data type.
Hash Table: An overview
Hash table is otherwise known as Hashmap. It’s a one data structure type that helps in storing the information through key-value pairs. The stored data in the Hash table can be retrieved with the help of the key as a reference. It’s the important reason why Hash tables are utilized as the look-up table data structure. This is due to the reliability and faster act during the storage of key-value pairs.
Hashmaps or Hash Tables in Python are implemented via the built-in data type. The keys of the built-in data type are generated with the help of a hashing function. The dictionary elements are not designed to be ordered and therefore they can be easily changed. Mapping of names of the student or employee with their Personal ID’s can be the best example of the built-in data type dictionary.
Learn Python from the Basic to Advanced Level with Hands-on Training, Placements, and more with
Python Training in Bangalore
Why Hash Tables or Hashmaps
Hashing is used to uniquely identify an object from a group of objects. In a hash table, each element is assigned a key/value pair where the key translates to a hash_value by making use of a hash function and then that hash function indicates where to add/delete/update the value. In python, we don’t need to dwell much in the hashing algorithm since it’s already implemented as dictionaries data structure here.
Before going into python implementation let’s look at why we need Hashing. Sometimes instead of sequential data structures, mapping data structures are required where it is easy to remember or find the key to access, update and delete the associated values and for a very large array it’s practically impossible to sequentially process each element and then find the value:
Example:
It’s comparatively easy to remember employee id that employee registration number,
Employee: Ramkumar: Name = Ram Lastname = Kumar Contact = xxxyyyzzzk
Hashtable Vs Hashmap
- Hash Table: They are fast, synchronized, and allows more than one null value and only one null key.
- Hash Map: They are slow, unsynchronized, and they do not allow null values or null keys.
How in Python
In python it’s implemented as dictionaries, In dictionaries, the items are stored as key-value pairs, where keys should be unique the reason for this unique requirement is this hashing in the background, the hash() function will collide if 2 keys will hash to the same value.
Note: Just for understanding we can take the example of memory address value, i.e., no two values can be stored at the same memory location at a time.
Get Placement Oriented Python Training from Industry Experts with our Python Training in Chennai
How to Create a Dictionary?
The built-in data type Dictionary can be created in two important ways namely
- With the help of dict() function
- With the help of Curly braces ({})
How to create a dictionary with dict() function?
dict() is the built-in function in Python, which is used to create dictionaries.
Let’s consider an example,
new_dict=dict() print(new_dict) type(new_dict)
OUTPUT:
{} dict
You can find an empty dictionary is created in the above example. The reason for this is that there are no key-value pairs are added as the parameter to the function dict().
You can also add values to the function dict() and this will look like the following example.
new_dict=dict(Viv = ‘001’, Bom= ‘002’) print(new_dict) type(new_dict)
Output:
{‘Viv’:’001’, ‘Bom’:’002’} Dict
How to create a dictionary with curly braces?
It’s easy to create a built-in function dictionary with the help of curly braces. Here is one best example of it.
Example:
1 my_dict={‘Viv’: ‘001’, ‘Bom’: ‘002} print(my_dict) type(my-dict)
The output of the above example will be as follows.
{‘Viv’:’001’, ‘Bom’:’002’} Dict
How to create Nested dictionaries?
Nested dictionaries are primary dictionaries which always lie within another dictionary. Let me explain this with a simple example.
student_details = {'Student': {'Viv': {'ID': '001', 'marks': 90, 'sex':'male'}, 'Bom': {'ID':'002', 'marks': 95, 'Designation': 'female'}}}}
How to perform Hash Tables operations with Dictionaries?
Below are some of the operations that can be used to perform on Python Hash Tables with dictionaries.
- Values updates
- Values access
- Deleting Element
Values updates:
Dictionaries are termed as mutable data types which indicate that updating them can be done whenever needed. Let me explain this with a neat example.
Let’s say that I need to change the student ID named Viv from ‘001’ to ‘003’ and also I need to add another pair of key-value to the dictionary, so it can be recorded as below.
Example:
my_dict={‘Viv’: ‘001’, ‘Bom’: ‘002’} my_dict[‘Viv’] = ‘003’ #value update My_dict[‘yus’] =’004’ #key-value addition print(my_dict)
Output:
{‘Viv’: ‘003’, ‘Bom’: 002, ‘Yus’: ‘004’}
Values Access:
The dictionary value is accessed in different ways namely
- With the help of key values
- With functions
- For loop implementation
Using Key values:
The values of the Dictionary are accessed with key values. Below is one example of it.
Example:
my_dict={‘Viv’: ‘001’, ‘Bom’: ‘002’} my_dict[‘Viv’]
Output:
‘001’
With Function:
There are different built-in functions namely get(), values(), keys(), and more.
Example:
my_dict={‘Viv’:’001’, Bom’ : ‘002’} print(my_dict.keys()) print(my_dict.values()) print(my_dict.get(‘Viv’))
Output:
dict_keys([‘Viv’, ‘Bom’]) dict_values([‘001’, ‘002’]) 001
For loop Implementation:
The loop enables you to access the dictionary’s key-value pairs by iterating over them. Let me explain this with an example.
my_dict={‘Viv’: ‘001’ , ‘Bom’: ‘002’} print(“All keys”) For a in my_dict: print(a) #print the keys Print (“all values) For a in my_dict.values(): print(a) #print values print(“all keys and values”) For a,b in my_dict.items(): print(a, “:”, b) #print keys and values
Output
All Keys Viv Bom All Values 001 002 All keys and values Viv:001 Bom_002
Learn Python Course to Boost your Carrer with our Python Online Training
How to delete items from dictionary?
There are many functions which enables you to delete information from a dictionary namely pop (), clear(), popitem(), del(), and more. Here is one of example.
Example:
my_dict{‘Viv’: ‘003’, ‘Bom’:’002’, ‘Yus’:’004’} Del my_dict[‘Viv’] #remove the pair of key values of ‘Viv’ my-dict.pop(‘Bom’) #removes the Bom value. my_dict.popitem() #remove the last inserted item print(my_dict)
Output:
{‘Yus’:’004’}
The example shows that every item except ‘Yus:004’ has been deleted from the dictionary with different functions.
How to convert a dictionary to the data frame?
We have already seen a nested dictionary example that contains students’ names and their information mapped to it. Now, let’s make use of the panda’s library to put them everything in a data frame.
Example:
Import pandas as ps Stu-details = {‘student’: {‘Viv’: {‘ID’: ‘001’, ‘marks’: 90, ‘sex’: ‘Male’}, ‘Bom’ : {‘ID’:’002’, marks’: 95, ;’sex’:’Female’}, ‘Yus’: {‘ID’:’003’,’marks’:’100’,’sex’:’male’}}} df=ps.DataFrame(stu_details[‘Student’]) print(df)
Output:
Viv | Bom | Yus | |
---|---|---|---|
Sex | Male | Female | Male |
ID | 1 | 2 | 3 |
Marks | 90 | 95 | 100 |
As a whole, let me describe the Hash table in short.
- It’s a group of key-value pairs
- There is no need for duplicate keys
- Hash table is otherwise called a map, associative array, dictionary
- 0(1) for get, delete, add functions
- We use dict as short for dictionary in Python
- The hashmap components include Array, which is a data structure that is used for storing the information. The hash functions are the function used for converting any type of key-value into an array index.
I hope the tutorial helped you to know about the hash tables and hashmap in-depth. Any queries? Ask us in the comment section below.