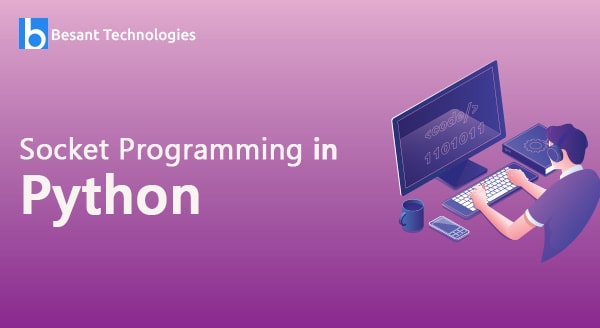
Socket Programming in Python
Socket Programming in Python
The activity of the internet is comprised of Networks and connections. The network functions with the help of the most critical Socket fundamentals. These networks are made conceivable utilizing one of the most vital basics of Sockets. This article covers all regions managing Socket Programming in Python. Attachments assist you with making these associations, while Python, without a doubt, and makes it simple.
What are sockets?
Sockets are inside endpoints worked for sending and receiving information. The network will have two Sockets, one for each communicating program or device. These sockets are combine with an IP address and a Port. A single device can have ‘n’ number of sockets dependent on the port number that is being utilized. Various ports are accessible for different protocols.
Why use sockets?
Sockets the foundation of networking. They make the exchange of data between two programs or devices. For instance, when you open up your browser, you as a customer are making a connecting with the server for the transfer of data.
- Did you ever realize that how you get Facebook notifications or Flipkart sale alert on your device.
- There is an end-to-end server setup working 24×7 to keep you acquainted with the latest updates.
- This client-server setup communicates with each other to get the updates.
What is the use of Sockets?
As defined above, the socket is considered to the network’s backbone. They help to make data transfer between two devices or programs efficiently. Let me explain this with an example. Say, you are opening your Google Chrome Or any other browser. You are now browsing for some queries over the internet. It means you are now the client who is creating a connection with the server for the information you need.
What is Socket in Python?
In simple terms, Sockets or Sockets programming is considered as the endpoint developed to send and receive the information. A single device or network has two sockets, and each one is derived for the respective communicating program or device. Sockets are also the integration of a Port and an IP address. Each of the device or program can have any number of sockets depending on the port number used. There are various ports available for different protocol types.
For example, here is a table that lists some of the typical port numbers and their relevant protocol.
Achieving Socket Programming in Python:
You should import a socket framework or module for making Socket programming in Python. There are built-in methods in these modules which are needed for building sockets. Below are a few methods with their descriptions.
- socket() – This method is used for creating sockets. It’s needed for both client and server ends to build sockets.
- accept() – This method is used for accepting a connection. It returns a different pair of values like conn, address. In this, the address is derived as socket address present at the other connection end, whereas conn is derived as the new object of the socket to send or receive data.
- bind()- It’s used for binding the address that is characterized as a parameter.
- close() – It’s used for marking socket as closed.
- connect() – It’s used for connecting to the remote address characterized as the parameter.
- listen() – It helps server to receive connections.
Learn Python from the Basic to Advanced Level with Hands-on Training, Placements, and more with
Python Training in Bangalore
Behind the scenes:
Entire connectivity between clients and servers is made possible via sockets. Sockets are nothing but the connectivity points between a client and a server. Just for example visualize the water supply connection from the water tank to your home, in this case, a water tank is the server, water is data and your house is a client. Now the joint between of water tank with the pipeline is the socket at the server-side, similarly joint of a pipeline with your home is the socket at the client-side.
Now coming to some technical example each application which you open which uses data is a client of some kind be it messaging application, social networking or carpooling what so ever it is, it’s a client for us. How they create sockets to get data/info will see later.
How Sockets work:
Since 1 socket is not sufficient for all the applications, therefore a device maintains 1 or more sockets per application and differentiate each 1 of them with a combination of ip_address and port_no. Some common port_no are given below:
- SMTP – 25. SMTP is known as the Simple Mail Transfer Protocol
- HTTP – 80. Port80 is associated with HTTP, Hypertext Transfer Protocol
- HTTPS – 443. HTTPS – 443 is also associated with the TCP protocol
- FTP – 20, 21
- TELNET 23
- IMAP 143
- RDP 3389
- SSH 22
Feel lucky as python is loaded with a very easy to use module for socket programming called ‘socket’
A glimpse of important socket methods is given below:
‘accept’, ‘bind’, ‘close’, ‘connect’, ‘connect_ex’, ‘detach’, ‘dup’, ‘family’, ‘fileno’, ‘get_inheritable’, ‘getblocking’, ‘getpeername’, ‘getsockname’, ‘getsockopt’, ‘gettimeout’, ‘ioctl’, ‘listen’, ‘makefile’, ‘proto’, ‘recv’, ‘recv_into’, ‘recvfrom’, ‘recvfrom_into’, ‘send’, ‘sendall’, ‘sendfile’, ‘sendto’, ‘set_inheritable’, ‘setblocking’, ‘setsockopt’, ‘settimeout’, ‘share’, ‘shutdown’, ‘timeout’, ‘type’
We will not dwell in details of all the methods rather will try with a simple example.
Server code:
A server code is nothing but a program, a computer, or a device that is given to overseeing network assets. Servers can either be on the similar device or connected to any devices and computers. There are different types of servers such as database, network and print servers, etc.
Servers generally make use of these techniques like socket.socket(), socket.bind(), socket.listen(), etc to establish a association and tie to the clients. Presently how to create program to create a server.
server.py
#!/usr/bin/python # This is server.py file import socket # Import socket module s = socket.socket() # Create a socket object host = socket.gethostname() # Get local machine name port = 55555 # Reserve a port for your service. s.bind((host, port)) # Bind to the port s.listen(5) # Now wait for client connection. while True: c, addr = s.accept() # Establish connection with client. i = c.recv(1024).decode('utf-8') print(int(i)) c.send(bytes(str(int(i)*4),'utf-8')) c.close() # Close the connection
Description:
Above code will receive a no. from client and multiply it with 4 and then send it to the client.
Client code:
A client code is nothing but software or computer programming that gets data or services from the server. In a client-server module, clients demands for services from servers. The right example is an internet browser, for example, Google Chrome, Firefox, and so forth. These internet browsers demand web servers for the necessary web pages and services as coordinated by the user. Different models includes web-based games, Chat, and so on.
Client’s needs to get some data from the server and for this, you have to utilize the recv() method and the data is stored in another variable msg. Simply remember that the data being passed will be in bytes and in the client in the above program can get up to 1024 bytes (support size) in transfer. It tends to be indicated to any amount of data will be transferred. Finally, the message being moved ought to be decoded and printed.
Since you know about how to make client-server programs, we should proceed onward to perceive how they should be executed.
client.py
#!/usr/bin/python # This is client.py file import socket # Import socket module import sys s = socket.socket() # Create a socket object host = socket.gethostname() # Get local machine name port = 55555 # Reserve a port for your service. s.connect((host, port)) s.send(bytes(sys.argv[1],'utf-8')) print(s.recv(1024).decode('utf-8')) s.close() # Close the socket when done
Description:
The above code will send a number which should be passed as command-line argument by the user, to the server and will receive the number from the server.
Note : We will not use any of the reserved port no in our cooked example, because that will conflict with the standard applications.
Output for server.py:
c:\users\amkum\Desktop> python server.py 28
Output for client.py:
c:\users\amkum\Desktop> python client.py 7 28 c:\users\amkum\Desktop>
Execute the programs, open the command, go into the folder which we have created your server and client program and then type :
py server.py (server.py is the filename of the server, py – 3.7 server.py)
When this is done, the server begins running. To execute the customer, open another cmd window, and type:
py client.py (client.py is the filename of the client)
How to create clients and servers for Socket Programming in Python?
Before going in-depth about the clients and servers creation for socket programming in Python, let’s have a basic understanding of what a client and a server is.
Client
A client is either a software or a computer that gets the services or information from the server. The client requests services from servers in case of a client-server module. Here is one example so that you can easily understand.
Example:
Web browsers like Firefox, Google Chrome, and more. The web browsers request the webserver for the web services and web pages as per the user direction. Some other example of the client is online chats, online games, etc.
Server
A server denotes either a device, computer, or a program that is biased to manage the network resources. There are chances for servers to be on the same computer or device or locally connected to other computers, devices, or even remote. Print servers, network servers, database servers, are some types of servers available. Servers utilize methods like socket.bind(), socket.socket(), socket.listen(), and more to make a connection and integrate the clients.
How to code the client-side program in Python?
Let’s consider the below example.
import socket s=socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.connect((socket.gethostname(), 2346)) msg=s.recv(1024) print(msg.decode("utf-8"))
Let me explain the above process point by point so that it can be more precise.
- I have imported the socket module.
- I am now creating a socket.socket() method to create a client-side socket.
- I am making use of the method connect() using the key pair values (host, port).
- I have used get hostname as the server, and the client is on the same device.
- I have used recv() as the client is required to get some information from the server.
- In the next step, it indicates that the data is stored in msg, which is another variable.
- As all we know, the data passed is in bytes, and therefore in the above example, the program can receive a maximum of 1024 bytes in just one transfer. It differs based on the data transferred
- At last, the message is transferred; they are decoded and then printed.
I hope you are clear about creating a client-side program in Python. Now, let’s move to the server-side application.
Get Placement Oriented Python Training from Industry Experts with our Python Training in Chennai
How to create a server-side program in Python?
Let me explain this with code as I did for the client-side program in Python so that you can understand clearly.
Example:
import socket s=socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.bind((socket.gethostname(),3456)) #anything between 0-65535 can be the port number (make sure to specify non-privileged ports that are > 1023) s.listen(7) while True: cln,adrr=s.accept() print(f"Connection to {adr}established") cln.send(bytes("Socket in Python","utf-8 ")) # send information to the clientsocket
- The primary step is that we are importing the socket module that we created.
- We are making use of the methods socket.socket() to create a server-side socket.
- I have used AF_INET; it means it’s the address from the internet, this needs (host, port) pairs. Here the host can be the website address or website URL, and the port number should be an integer.
- I have made use of the SOCK_STREAM to create TCP protocols.
- The method bind() is capable of accepting two parameters as a tuple like pairs (host, port). I’m using a four-digit port number because it’s easy for the lower ones to occupy soon.
- I have made use of the method to listen() to enable the server to accept connections.
- Here, 7 denotes the queue for different connection that pops alternatively.
- 0 is the minimum value specified, and if you give any less value, it automatically gets changed to 0. If you are not providing any parameters, then it takes the default value.
- The while loop indicates that you accept connections always.
- I have denoted the client object and address as ‘cln’ and ‘adrr.’
- The print statement used is used to print the socket port number and address.
- At last, I have used cln.send to send the data in bytes.
Got any queries? Let us know in the comment section below. To get more knowledge on the Python and its applications, you can start enrolling in the Python Online training from us. Contact us for more details.
Learn Python Course to Boost your Career with our Python Online Training