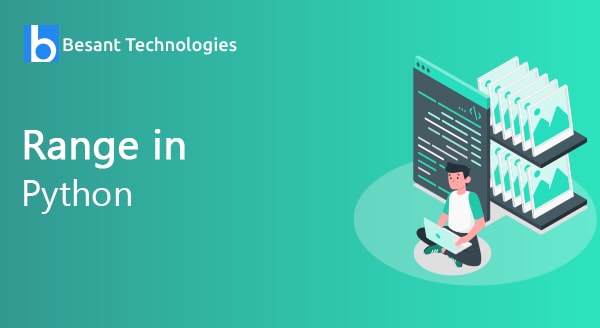
Range in Python
In python we have different data types like list, tuple and etc.. Like that we have another built-in function called range (). This function is mostly used in looping statements. It also used to store the sequence of numbers specified in the function parameter.
It is an inbuilt function to generate the sequence of numbers that starts with specified parameter values. The default value is from 0 to increment the values by 1 when you are not given any stop values. It is mostly used in loops.
Syntax:
range (start, stop, step)
start – It is an optional parameter. It is also used to start the sequence numbers from a specified value. It is also a starting parameter. If the start parameter not given sequence starts with 0.
stop – It is a mandatory parameter. Whereas other parameters are not specified not a problem but must specify the stop parameter. It is used to stop the sequence of numbers at a specified value.
step – it is an optional parameter. It is used to iterate (Increment or decrement) the sequence with specified step values. It not given value default is 1.
Note: stop value is not considered. It is taken as only an end, not in sequence. Sequence stoped before stop value. i.e range(5) means it will take up to 5. 0 to 4.
Example:
for i in range(5): print(i)
Output:
0 1 2 3 4
In this example, we use the range function with a stop value of 5. Also, implement the range inside for loop. It’s only specified the stop value itself so that the sequence starts with 0. Step also not used so that it will increment the value by 1.
Example:
for i in range(2,5): print(i)
Output:
2 3 4
In this example, we use the two parameters called start and stop as 2,5 respectively. Here also we are not providing steps so that it will increment the value up to the stop number.
Example:
for i in range(1,5,2): print(i)
Output:
1 3
In this example, we use all parameters of the range method. Here the range will take 1 as a start, 5 as stop and 2 as step value to generate the sequence of numbers.
Example:
for i in range(6,0,-1): print(i)
Output:
6 5 4 3 2 1
In this example, we use the reverse process of range function with start with 6. Stop at 0 with a step of -1. It is also called a negative process in range function.
Range vs Xrange:
In python we used two different types of range methods here the following are the differences between these two methods.
- range() method used in python 3.x. whereas xrange() used in python 2.x.
- The functionality of these methods is the same.
- Here the range() will return the output sequence numbers are in the list. But the xrange() will return it as xrange object. i.e generator object.
- Range() methods takes more memory to store values because it returns the output as a list of values. Whereas xrange() consumes less memory because it will return only xrange object.
- The manipulation process in range() is easily done because it returns the list of values. But it cannot be done in xrange(). Because it returns xrange object. It is also a disadvantage of xrange() method.
- Using of generator object xrange() is faster than range() for implementation.
Concatenate ranges:
Example:
from itertools import chain a=chain(range(5), range(6,10)) for i in a: print(i)
Output:
0,1,2,3,4,6,7,8,9
In this example, we implement chain concept. Here it used to concatenate the two range elements. Not like adding the elements it just adds the elements at the end of first range. In this example, the first range returns the value of 0 to 4 and second one added with this range with 6 to 9.
Accessing Values of range
Example:
first=range(0,5)[2] second=range(0,5)[4] print(first) print(second)
Output:
2 4
In this example, we use to access the elements or values in the range using the normal indexing process.
Convert range into list
Example:
c=range(5) d=list(c) print(d)
In this example, we just use the range function inside the list constructor to convert the sequence of values into a list of elements.
Output:
[0,1,2,3,4]
Important Points about range:
- In range only return the integer values it not allowed other types of values.
- Arguments of the range also be an integer value.
- All the arguments are either positive or negative integers.
- It is also a data type in python.
- Step argument value cannot be zero. If you pass 0 it will throw traceback error ValueError.
- Access the elements using an index.