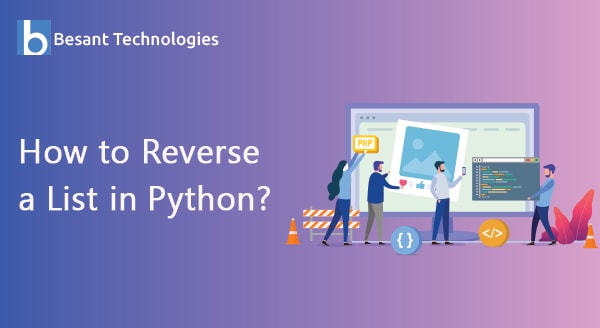
How to Reverse a List in Python
Reverse a list in Python
In Python, we have different types of container classes. Now, this tutorial we discuss the list. Especially reverse the elements of the list. In List is a mutable container class and it also contains duplicates. So it allows to manipulate the objects. In that manipulation, we going to discuss reversing the objects like a stack to queue format.
Reversing the list is done in python using the reverse () method in the list class. It just reverses the list based on the index values. It’s not created and copy the new list just using the existing list and reverse the objects in the same list itself.
Example:
>>> l=[1,2,3,4,5] >>> print(l) [1, 2, 3, 4, 5] >>> l.reverse () >>> print(l) [5, 4, 3, 2, 1]
In this example, we add some elements in the list and call the reverse() method. It just reverses the elements based on their positions.
Example:
>>> l1=[1,2.5,"hello"] >>> print(l1) [1, 2.5, 'hello'] >>> l1.reverse() >>> print(l1) ['hello', 2.5, 1]
In this Example also we reverse the list elements. Why we see this example because somewhat have doubted whether we are using the same datatype for reversing but its no need to maintain some type of values because of its only reverse elements based on an index, not ASCII.
Example:
>>> l2=["one","two","three","four","five"] >>> print(l2.reverse ()) None
In this Example reverse() method not reversing the elements. It returns None because it cannot reverse the large sequences. Because while reversing the list in economy space that is average of memory space so that it returns None instead of a reverse list. Moreover reversing the list having some advantages and disadvantages.
The advantage of the reversing list using the reverse() method is working very fast and shuffle the elements of a list without creating any new list. So it saves memory.
The disadvantage is changed the original list so that we need to again use the reverse() method to get the original state.
Example:
def reverse_list(list): print('before reverse :', list) list.reverse() print('after reverse:', list) num_list = [1,2,3,4,5] str_list = ["six", "seven", "eight", "nine", "ten"] reverse_list(num_list) reverse_list(str_list)
In this example, we overcome the previous example output None. Here we are using a separate user-defined method with passing the list of elements as a parameter and pass the list of elements. Also, reverse the elements inside the function.
Output:
before reverse : [1, 2, 3, 4, 5] after reverse: [5, 4, 3, 2, 1] before reverse : ['six', 'seven', 'eight', 'nine', 'ten'] after reverse: ['ten', 'nine', 'eight', 'seven', 'six']
Reverse a list Using Slicing:
It is also possible to reverse the list without using the reverse() method. That will be done by using the slicing technique. Here we can use the reverse indexing to achieve this.
Example:
def reverse_list(list): rev_list = list[::-1] print('before reverse:', list) print('after reverse:', rev_list) num_list = [1,2,3,4,5] str_list = ["six", "seven", "eight", "nine", "ten"] reverse_list(num_list) reverse_list(str_list)
Output:
before reverse: [1, 2, 3, 4, 5] after reverse: [5, 4, 3, 2, 1] before reverse: ['six', 'seven', 'eight', 'nine', 'ten'] after reverse: ['ten', 'nine', 'eight', 'seven', 'six']
In this slicing process for reverse, a list will be a copy of the list of elements. So that it will take more memory when compared to the reverse () method. Also, it creates a copy so that it holds more space for the existing elements of the list.
Here we note the point whether the structure of the list is copied not contained objects. i.e elements of the list. Hence it no needs for extra memory because elements are not duplicated. It also holds the address of the list elements updated to the structure of the reference. Slicing is fast but the code understanding is quite difficult.
Reverse the list using reversed() function:
In this function, it will return the Iterator form of reverse. So we can access the values in reverse order. It does not reverse a list instead of it will create a new copy of the list. Also, it will access individual elements to reverse order.
Example:
def reverse_list(list): reversed_list = [] for o in reversed(list): reversed_list.append(o) print('before reverse:', list) print('after reverse:', reversed_list) num_list = [1,2,3,4,5] str_list = ["six", "seven", "eight", "nine", "ten"] reverse_list(num_list) reverse_list(str_list)
Output:
before reverse: [1, 2, 3, 4, 5] after reverse: [5, 4, 3, 2, 1] before reverse: ['six', 'seven', 'eight', 'nine', 'ten'] after reverse: ['ten', 'nine', 'eight', 'seven', 'six']
In this example, we have done the reversed() method format to reverse the elements of the list. It is an Iterator pattern too. So all the elements of the list traversed into reverse order.
Example:
>>> a=[1,2,3,4,5] >>> print(reversed(a)) <list_reverseiterator object at 0x02B24550> >>> print(list(reversed(a))) [5, 4, 3, 2, 1]
This example is another way to use reverse the list. Here we are using reversed() method inside the list constructor. The list constructor has iterated the list up to the last element of the list to get the reverse order but it will also generate the shallow copy of the list.