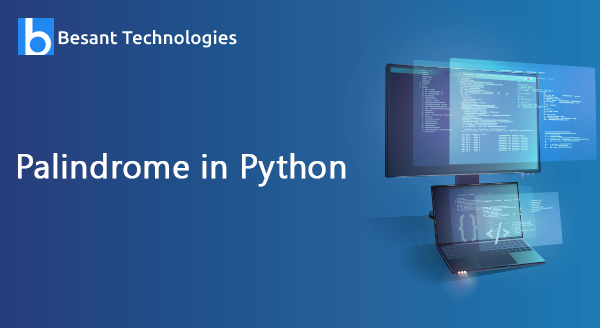
Palindrome in Python
A string or a number is a palindrome if the reverse of the said number or string is the same as the string. For example, 101 and madams are number and string palindrome respectively. We will go through some methods to check if a string or a number is a palindrome or not
Method 1
- Create the reverse of the string or the number
- Now check if the reverse of the string is the same as the original string.
def palindrome_checker(string): #Storing the reverse of the string in rev rev_string = string[::-1] #Check if both strings are equal or not if (string == rev_string): return True return False s = "madam" ans = palindrome_checker(s) print(ans)
Method 2
In this implementation of palindrome_checker, if there are n characters in a word, we will compare the 1st character to the last one, 2nd character to the second last character and so on. If any character mismatches then it cannot be considered as a palindrome.
Below is the implementation of the above approach that has been explained:
import numpy as npdef palindrome_checker(string): for index in range(0, int(np.ceil(len(string)/2))): if string[index] != string[len(string)-index-1]: return False return True s = "madam" ans = palindrome_checker (s) print(ans)
Method 3
Using join and reversed functions which
def palindrome_checker(string): rev_string = ''.join(reversed(string)) if (string == rev_string): return True return False s = "madam" ans = palindrome_checker(s) print(ans)