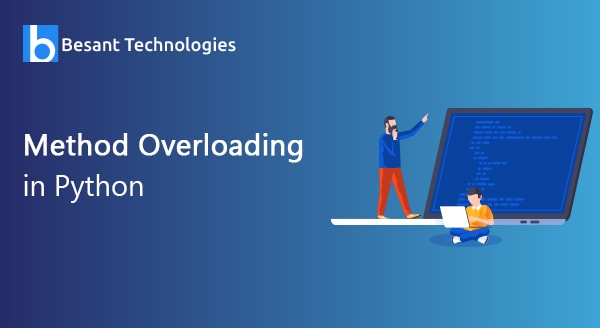
Method Overloading in Python
What is Method Overloading?
In oops we have different types of elements like Object, Class and etc. in these elements we implement the reusability in tow elements are Inheritance and Polymorphism. In this topic, Method overloading is in the Polymorphism. Polymorphism is a technique of producing different functionality with a single format.
Here we have two types of polymorphism.
- Method Overloading
- Method Overriding
Method Overloading:
-
- Method Overloading is the class having methods that are the same name with different arguments.
- Arguments different will be based on a number of arguments and types of arguments.
- It is used in a single class.
- It is also used to write the code clarity as well as reduce complexity.
Method Overriding:
- Method Overriding is the method having the same name with the same arguments.
- It is implemented with inheritance also.
- It mostly used for memory reducing processes.
Learn Python from the Basic to Advanced Level with Hands-on Training, Placements, and more with
Python Training in Bangalore
Method Overloading in Python
Method overloading is one concept of Polymorphism. It comes under the elements of OOPS. It is actually a compile-time polymorphism. It is worked in the same method names and different arguments. Here in Python also supports oops concepts. But it is not oops based language. It also supports this method overloading also.
Normally in python, we don’t have the same names for different methods. But overloading is a method or operator to do the different functionalities with the same name. i.e methods differ their parameters to pass to the methods whereas operators have differed their operand.
Here some advantages of Method Overloading in Python.
- Normally methods are used to reduce complexity. Method overloading is even it reduce more complexity in the program also improves the clarity of code.
- It is also used for reusability.
It has some disadvantages also when creating more confusion during the inheritance concepts.
In python, we create a single method with different arguments to process the Method Overloading. Here we create a method with zero or more parameters and also define the methods based on the number of parameters.
Example:
class Employee: def Hello_Emp(self,e_name=None): if e_name is not None: print("Hello "+e_name) else: print("Hello ") emp1=Employee() emp1.Hello_Emp() emp1.Hello_Emp("Besant")
In this example class called Employee having a method called Hello_Emp(). This method is used as a method overloading concept. It will be done by using the parameters. Here we use the parameter called e_name is set as None. If I call this method using objects during that time if I pass the value to the parameter means it prints the value. Otherwise, it is not print anything. So here we have two functionalities that will be executed in this program.
Output:
Hello Hello Besant
In this output, we first call the method Hello_Emp without any parameter so that it prints only Hello.
Next time we call the same method with parameter value as besant so it will prints Hello Besant.
Get Placement Oriented Python Training from Industry Experts with our Python Training in Chennai
Example:
class Area: def find_area(self,a=None,b=None): if a!=None and b!=None: print("Area of Rectangle:",(a*b)) elif a!=None: print("Area of square:",(a*a)) else: print("Nothing to find") obj1=Area() obj1.find_area() obj1.find_area(10) obj1.find_area(10,20)
Output:
Nothing to find Area of a square: 100 Area of Rectangle: 200
In this example also we implement the Method Overloading concept with help of Parameters. In this example use to find the area of different shapes. If you get the area of square means you just pass the value for the parameter itself. In the same method if you want to find a rectangle area means you pass the value for both a and b values. If you pass without any value means it will return nothing to find.
Learn Python Course to Boost your Carrer with our Python Online Training