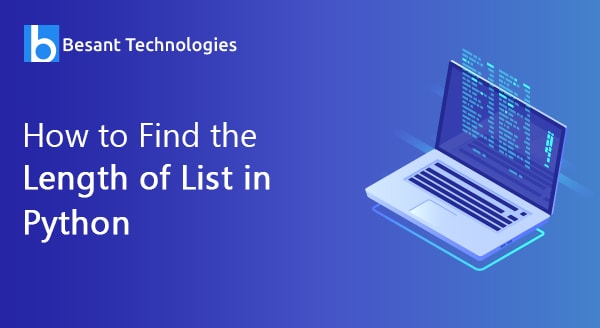
How to find length of list in python
Length of list in Python
List is a collection of elements with duplicates. It is also called ordered collection i.e what order you insertion the elements the same order it will return. It also follows the index-based storage so that the elements are arranged in order. It is also needed not to be homogeneous i.e storing similar types of values. But in a list you can store different types of elements also. It is mutable also we can able to manipulate the elements like adding, removing and changing the values.
Example:
l1 = ['besant', 'python', 2019] l2 = [1, 2, 3, 4, 5,6,7,8 ]; l3 = ["a", 2,10.4]
In this tutorial, we discuss finding the length of the list. i.e number of elements in a list. To find the length of the list we can have two ways.
- Using predefined method len(list)
- Using user defined function (logic).
Using len() Method
This method is commonly used to finding the length of the list, tuple, string and etc also. It will take the object of the container data types and return the number of elements present in the container data type.
This len() method is the easiest and convenient method for finding a number of elements in python.
Syntax:
len(data type) for List len(list)
Here the list is the object of the list.
Example:
l=[1,2.5,'hello'] print(l) print(“Number of Elements:”,len(l))
Output:
[1, 2.5, 'hello'] Number of Elements:3
In this example list, l will have different types of elements. Now we use the len() method to find the number of elements in the list. Just passing the object as a parameter to find which list wants length and get the result integer for the count.
Using a User-defined function:
In this, we can create a user-defined method to find the length of the list. It also uses the looping process to get the number of elements.
Example:
l=[1,2.5,'hello'] count=0 for i in l: count=count+1 print("List:",l) print("Number of Elements:",count)
In this user-defined method, we use the count variable to find the number of elements in the list. Here also we used for loop to do this. It is technic of using collection controlled loop format (for). In this collection, controlled loops are not following any condition it follows only the length of the collection. For that, we just add the value of count by 1. And finally, it returns the number of elements.
Output:
List: [1, 2.5, 'hello'] Number of Elements: 3