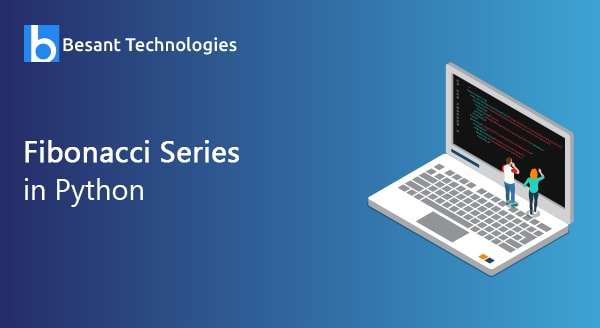
Fibonacci Series in Python
Python is a general-purpose programming language with the process of interpreted, high-level programming language. It also supports OOPS concepts. It mainly used to generate standard software applications. Understanding the concepts of python is helps us to create different types of Applications using python. For that, we use some fundamental programs to do it. That we also called implement the logic process in programs like finding prime no, even or odd no’s and etc..
What is the Fibonacci Series?
Fibonacci the Italian mathematician found this logic. It is a sequence of numbers. The sequence will start with 0 and 1. And the sequence will follow the addition of these two numbers. It simply given the third number will be the addition of the previous two numbers.
Example:
0, 1, 1, 2, 3, 5… this is an example of the Fibonacci series the current number will be the addition of the previous two numbers. Here the first two numbers is 0 and 1 respectively. The third number is 1. It will come by 0+1=1. The next number also comes like 1+1=2. This is a way it produces the results up to n.
Now see the examples to implement this Fibonacci series in python.
In python, we implement this Fibonacci series in two simplest ways as following
- Using a Loop
- Using a Recursive function
Fibonacci Series using a Loop:
Example:
# Enter number of terms needed #0,1,1,2,3,5.... n=int(input("Enter the n terms")) fir=0 #first element of series sec=1 #second element of series if n<=0: print("The fibonacci series is",fir) else: print(fir,sec,end=" ") for x in range(2,n): next=fir+sec print(next,end=" ") fir=sec sec=next
In this example, we implement the Fibonacci series. First, we will take the input as n for a number of the sequence we need and then we declare first and second values as 0 and 1 respectively because of these values the default of starts the Fibonacci series. Then we implement the logic with if the condition of n<=0. If the n is 0 means it takes the first itself as a result. The else we use for with range to process the sequence. Set the stop value of the range as n. then implement the swapping concept to interchange the values. i.e first and second will be changed after every iteration. Then the addition of first and second will stored in next.
Output:
Enter the n terms 5 0 1 1 2 3
Fibonacci series using the recursive function:
A recursive function is a process of calling the function by itself directly or indirectly. Using this recursive function some logic programs will be done easily. Mostly repeated processes of logic programs is using this function.
Example:
def Fib_Recur(a): if a <= 1: return a else: return(Fib_Recur(a-1) + Fib_Recur(a-2)) n = int(input("Enter the no.of.terms? ")) # take input from the user if n <= 0: # check if the number is valid print("enter the positive values only ") else: print("Fibonacci Series:") for i in range(n): print(Fib_Recur(i))
In this example, we implement the Fibonacci series using a recursive function called Fib_Recur. Just pass the no.of terms as an input and returns the result of the current value with additional previous values as a recursive process. Here also take input and check the <=0. i.e positive value and call the recursive using for loop with range function. But here use only stop value in range.
Output:
Enter the no.of.terms? 6 Fibonacci series: 0 1 1 2 3 5