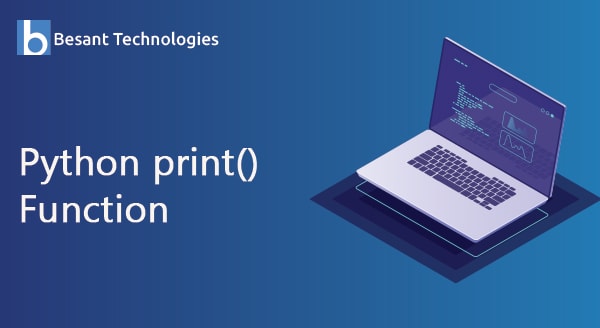
Python print() Function
Python print() Function
In all the programming languages having some standard functions to print the values in console or store values in the file. Because printing the values in programming is a fundamental thing for output. In python is simple to process the outputs using the print function.
Normally a print function is nothing but process the output or result to display in the console. It is also a standard function.
Syntax:
print(values ,sep=’’ , end=’\n’, file=file, flush=flush)
Parameter Values
values – Sequence of values to print. It may any type of value. Also, convert string before print.
sep (separators) – used to separate the values if its more than one.default is ‘ ‘. Also optional.
End – (end) print the object or value at the end. Default is ‘\n’.Also optional.
file=file – write a method for writing objects. Also optional.
flush=flush – if true, specify the buffered flush otherwise false. Default is False. Also optional
Example:
print(“This is Python”)
In this example print function without optional parameters. Here we simply print the value or object as we require. Here we just print the string to the console.
Example:
a=10 b=’world’ print(“Hello”,a,b)
Output:
Hello10world
In this example, we print more than one value or object in the console. Here the more than values may any type but it will convert into a string and also values or objects are separated by a comma.
Example:
a=” hello” b=” world” print(a+b)
Output:
Helloworld
In this example also we concatenate the values or objects and print as a string.
print with Separators
separators are used to create partitions the objects into different in the print statement. The default is (‘ ‘) whitespace as used by this attribute. Also, the user allowed to change the value of this attribute when it’s required.
Example:
a=10 b=”welcome” c=2020 print(a,b,c,sep=’,’)
Output:
10,welcome,2020
In this example, we are using a separator as ‘,’ comma for differentiating the objects. Normally separator follows the whitespace(‘ ‘) to separate objects but here we are using ‘,’ rather than whitespace.
print with end parameter
if you try to print the objects without using end means it will print in the separate lines of the element.
Example:
a=”hello” b=”world” print(a) print(b)
Output:
hello world
In this example, e are print the values a and b in print function. The output will come in two lines of statement because we are not using the attribute. Now, you see how to print the values using end attributes in the e line.
Example:
a=’hello’ b=’world’ print(a, end=’&’) print(b)
Output:
hello & world
In this example, we are using the end attribute as ‘&’ symbol. So that the values of the same a and b in the previous example will print in the same line of the statement. It’s a use of end optional parameter in the print statement to print different objects in a single line.
print with file attribute
It is also we can print the different types of objects into the file using the ‘file’ attribute. Just pass the path of the file in print statement. I suppose the file is not created or the present print function creates a new file and writes it in the file.
For this attribute, we need an open function to locate the file for writing.
f=open(path of file, mode).
Here the path is an exact location if a file and mode are read(r), write(w) and append(a). but for our print function, we are using write mode only.
Example:
f=open(“one.txt”,”w”) print(“Hello world”,file=f) f.close()
Output:
Hello world will be stored in one.txt file.
In this example, we print the values in the text file instead of using the console. This will be done by using the attribute file. In other languages its called print-stream process.
print with flush parameter
now we going to implement the flush attribute in the print function. Its used to print the different objects with buffered or unbuffered output. The default value of this optional parameter is False. If it is False means its buffered the output. If it is True means unbuffered the output and also working quite slowly comparing with normal flow.
Example:
import time f=open("received.txt","r") start=f.read() sttime=time.time() print(start,flush=False) ettime=time.time() print(ettime-sttime)
Output:
Hello World 0.012000083923339844
In this example, we read the data from the file with the flush attribute value of False. It seems to be a time duration of 0.01200083923339844 secs. It is calculated using the start time and end time of the whole reading process.
Example:
import time f=open("received.txt","r") start=f.read() sttime=time.time() print(start,flush=True) ettime=time.time() print(ettime-sttime)
Output:
Hello World 0.01500082015991211
In this example see the time duration of the reading process is 0.01500082015991211 is taken more time when comparing to the previous reading example. Because of here we are using the flush attribute value as True. So we discuss earlier if you are using flush=True means it will slower than the normal flow.
Function in Python
Function is a set of instruction that gets executed once it is being called. Function defines the scope of the object and creates the line of execution.
There are two phase in which function is used:
- Function Definition
- Function Execution
Function Definition
Here function is defined and loaded into the memory.
To define the function, following syntax is used
def <functionName> :
# code block
Function Execution
This will call the function and execute the instructions written inside the function. A function will never get executed until it is called.
To call a function, one need to use function name followed by parenthesis.
Example:
Function Definition
def add() :print ("hello") print ("my function")
Function Execution
add()
Output:
hello my function
Types of Function
- Default Function
- Parameterized Functions
- Function with Reaturn Types
- Optional Parameter
Default Functions
This function definition just contains a set of instruction defined inside a function.
Parameterized Function
This function is defined with set of instruction that takes number of data (arguments / parameters) from outside and use that inside the block. Basically, one can pass data to the function.
Example:
def add (a,b):
print(“hello”)
print(a+b)
output:
>>add (3,4) hello7
Function with return type
In this function definition, data can be thrown outside the function scope. Usually anything that is declared inside the function cannot be accessed outside the function. Using ‘return’ keyword one can identify what specific data can be accessed.
Example:
>>def add (a,b) : return a+b >>> res=add (3,4) >>> print(res) 7
Optional Parameter
In this function definition, one can set the weather parameter needs to be optional or mandatory by defining a default value against the parameter.
Example:
>>def add(a,b)=4print (a+b)>> (add 3)7 >> add (3,10) 13
Here ‘b’ is an optional parameter so if the value of ‘b’ is not passed while calling the function, a default value of 3 will be considered.
Advantages
- Makes code loosely coupled.
- Remove Code Duplicate problem
- It makes code clearer and more understandable.
Python Print()
The print is a built-in function in python which lets you print show objects. The object that you pass on to print is first converted to a string before being cast on to the screen. The print is also the hello world program that usually launches someone in the amazing world of python!
In this tutorial, we will learn –
- Basic print syntax
- Using the print syntax
- Printing multiple statements
- Using new line characters
- Printing ‘ in a string
- Other Parameters in print
Below is the print syntax with different parameters that can be used inside it.
print(value(s), sep= ' ', end = '\n', file=file, flush=flush)
The print statement has a very simple format. At the very least, it takes nothing and prints an empty string. ‘sep’ parameter stands for the separator. This parameter decides, if there are multiple statements what should the resultant printed statements be concatenated by. The default is ‘ ’ or space. The ‘end’ parameter is used to write a statement towards the end of the printed statement. The default value is ‘\n’ or a new line. ‘file’ parameter is an object with a write method. This is used to write the print statement using the object. The default for this parameter is the standard output. The final parameter is the ‘flush’ parameter. This is a Boolean value that tells if the output is buffered which is denoted by False and flushed is denoted True. The default for this value is False.
2.Using the print syntax
The print statement has a really easy statement to use. Below is the basic python usage –
Input:
print('Hello World!!!')
Output
Hello World!!!
This amazing piece of code will print the words “Hello World!!!” to your screen. Amazing right? One thing to note is that in print statements as in python single quotes(‘) and double quotes(“) are interchangeable, But both of the opening quotes and closing quotes should be the same.
3. Printing multiple statements
As easy as it is to print a single statement in python, the same statement can be used to print multiple statements at once. The below statement prints out multiple statements –
Input
print('Hello World!!!','I am learning python.','It is really amazing!')
Output
Hello World!!! I am learning python. It is really amazing!
In the above code, we will get the entire statement separated by a space which is the ‘sep’ parameter is the print statement that we discussed earlier. The same syntax can be used to print numbers and strings together! Below code shows you how –
Input
print('Hello World!!!','I have been learning python for',4,'hours now.')
Output
Hello World!!! I have been learning python for 4 hours now.
See in the above syntax we printed both strings and numbers together.
4. Using new line characters
You can also use characters that have a special meaning attached to it. For example a new line character(\n) or a tab(\t). When using \n in a string and printing it, it results in an actual new line in the print output.
Input
print('Hello World!!!','\nI am in the new line!')
Output
Hello World!!! I am in the new line!
In the above code, the new line gets converted to an actual new line in the print output!
5. Printing ‘ in a string
In English, there are a lot of words like L’oreal which has a single quote inside it. When printing it on the console, it will throw a syntax error if used with a single quote. In order to prevent the error there 2 options –
print('L\'Oreal') print("L'Oreal")
In the first method, we use \ so that we escape the importance of ‘. In the second option, the entire text is encapsulated in “ while we have an ‘ in the text.
6. Other Parameters in print
Let’s see a few examples where we use the other parameters.
Input
print('Hello',end='@') print('World')
Output
Hello@World
Input
print('Hello World!!!','I have been learning python for',4,'hours now.', sep='$')
Output
Hello World!!!$I have been learning python for$4$hours now.