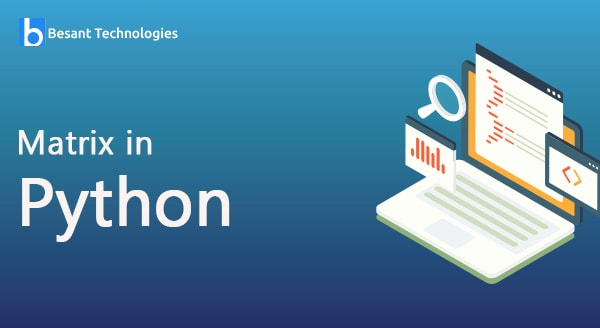
Matrix in Python
Matrix Introduction
Matrix is a mathematical term for arranging numbers and symbols in a rectangular format like rows and columns. formally in software languages, it is called a two-dimensional array of a data structure for storing values in rows and columns.
for ex: a matrix with 2×3 means 2 rows and 3 columns of data totally 6 values (2*3=6).
How to use the Matrix in Python
Not only in python all other languages also not having specific methods or classes for handling matrix formats. this kind of format is used via array concepts.
in python used the list or n array concepts are used for matrix processing.
- the list is used to store different types of values
- nd array is used in numpy.
Matrix with the list
here the matrix creating using list of the list.
Example:
mat=[[1,2,3], [4,5,6]]
its an example of a 2×3 matrix. we have two lists with every three values stored in another list.
let us see how to processing matrix values
mat=[[1,2,3],[4,5,6]] for i in range(2): print("row:",i) for j in range(3): print(mat[i][j]) print("mat=",mat)# total list print("mat[0]=",mat[0])#first row print("mat[0][2]=",mat[0][2])# getting specific element print("mat[1][-1]=",mat[1][-1]) # last element in second row
Output:
row: 0 1 2 3 row: 1 4 5 6 mat= [[1, 2, 3], [4, 5, 6]] mat[0]= [1, 2, 3] mat[0][2]= 3 mat[1][-1]= 6
Matrix with nd-array (numpy)
numpy is a module used for creating powerful n-dimensional array objects.
note: you need to install numpy module for use it. (pip install numpy)
Let see how to process matrix in ndarray
import numpy as np mat = np.array[[1,2,3],[4,5,6]] print("mat=",mat) mat_zero=np.zeros((2,3)) print(mat_zero) mat_shape=np.arange(6).reshape(2,3) print(“mat_shape=”,mat_shape)
Output:
mat = [[1 2 3] [4 5 6]] [[0. 0. 0.] [0. 0. 0.]] mat_shape = [[0,1,2] [3,4,5]]
Matrix Manipulation
Addition of two matrices:
Addition two matrices are mat1 and mat2 gets the value of mat3. for a better understanding of the matrix program, we need knowledge about looping (for) and list.
mat1 = [[1,2,3],[4,5,6]] mat2 = [[1,2,3],[4,5,6]] mat3 = [[0,0,0],[0,0,0]] for i in range(2): for j in range(3): mat3[i][j]=mat1[i][j]+mat2[i][j] print("mat1=",mat1) print("mat2=",mat2) print("mat3=(mat1+mat2)",mat3)
Output:
mat1= [[1, 2, 3], [4, 5, 6]] mat2= [[1, 2, 3], [4, 5, 6]] mat3=(mat1+mat2) [[2, 4, 6], [8, 10, 12]]
Transpose of the Matrix:
In this example, we interchange the rows and columns of mat1 into mat2. i.e 2×3 matrix will be converted into a 3×2 matrix.
mat1= [[1,2,3],[4,5,6]] mat2= [[0,0],[0,0],[0,0]] for i in range(len(mat1)): for j in range(len(mat1[0])): mat2[j][i]=mat1[i][j] print(“mat1=”,mat1) for i in mat2: print(i)
Output:
mat1= [[1, 2, 3], [4, 5, 6]] [1, 4] [2, 5] [3, 6]
Matrix Multiplication
In this example did the matrix multiplication. the rule of matrix multiplication is mat1 columns is equal to mat2 rows values. the result will be the format of mat2.
For example :
mat1 is 2×3 means mat2 will be 3×2. the result is the same as mat2.
mat1=[[1,2,3],[4,5,6]] # 2X3 matrix mat2=[[1,2],[3,4],[5,6]] # 3X2 matrix for rule of matrix multiplication mat3=[[0,0],[0,0],[0,0]] for i in range(len(mat1)): for j in range(len(mat2[0])): for k in range(len(mat2)): mat3[i][j]=mat3[i][j]+(mat1[i][k]*mat2[k][j]) print("mat1=",mat1) print("mat2=",mat2) print("mat3=") for i in mat3: print(i)
Output:
mat1= [[1, 2, 3], [4, 5, 6]] mat2= [[1, 2], [3, 4], [5, 6]] mat3= [22, 28] [49, 64] [0, 0]
Related Blogs:
- Brief Overview of Python Language
- Python Career opportunities
- Python Break Continue
- Python Control Flow
- Python Data Types
- Python Dictionary
- Python Exception Handling
- Python File
- Python Functions
- Python Substring