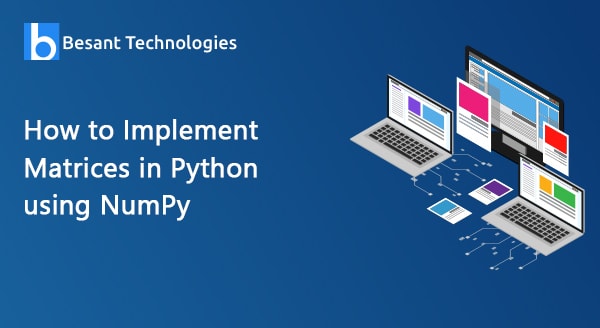
How to Implement Matrices in Python using NumPy?
Matrices can be used as a useful mathematical tool for different purposes in the real world. In this tutorial, we will discuss implementing those matrices in Python with the help of NumPy in depth.
NumPY | An overview:
NumPy is one type of Python libraries that enables easy numerical calculations. It involves single as well as multidimensional matrices and arrays. Based on the name, it shines in making mathematical calculations. There are different data science libraries in the world that depend on NumPy, namely Scikit-learn, matplotlib, SciPy, Pandas, and more. It acts as an essential part of the present data science applications which are using Python.
With the help of NumPy, it’s easy to solve many linear algebra calculations. The core mathematical tool which is utilized in different machine learning algorithms is Linear algebra. Knowing about Numby will help you to extend the current machine learning libraries and to create libraries.
NumPy offers
- Broadcasting functions
- Useful Fourier transform, linear algebra, and capabilities in the random number
- Tools for integrating Fortran code and C/C++
- A practical N-dimension array object defined as ndarray.
Let’s check out how to create a matrix in NumPy in depth below.
Making use of lists to create a matrix
## Import numpy import numpy as nm ## Creating a 2D numpy array with the help of python lists ary = nm.array([[ 4,5,6],[ 7,8,9]]) print(ary)
Nm.array is used for creating a NumPy array from a list. The output of the above program will be.It indicates a 2D matrix in which the input to nm.array() is a list of lists [[ 4,5,6],[ 7,8,9]].
The output will be
Using ranges to create a matrix
nm.array() can generate a number sequence provided with start and end.
## Generate numbers from (start) to (end-1) ## Here start = 1 ## end = 6 ## Generated a NumPy array from 1 to 5 print(nm.arange(1,6))
The output of the above 1D array will be
If you need to generate the same in 2D matric, then you can make use of the nm.arange() within a list. We will pass this list to the nm.array() that makes it look like a 2D NumPy array.
print(nm.array([nm.arange(1,6), nm.arange(6,11)]))
The output of the above array will look like
NumPy array shape
The array of N-dimensions is used to refer to any of the NumPy objects. It is indicated as a matrix of N-dimension in mathematics. Each of the NumPy objects of ndarray is queries based on its shape. n_rows,n_cols is the shape, which is a format tuple. The snippets below are used for printing the matrix shape.
arr_2d = nm.array([nm.arange(1,6), nm.arange(6,11)]) print(arr_2d.shape)
The output of the above snippets will be (2,5) indicates that the matrix holds two rows and five columns.
(2,5)
Let’s check out the example for the matrix that is filled with ones and zeros.
Filled with zeros:
## Create a matrix of shape (5, 6) filled with zeros
## By default we have specified it as float64 as the type of numbers are generated if not indicated
print(nm.zeros((5, 6)))
Output:
By default, the generated type of numbers will be in the array float64 if not indicated.
Let’s now check the matrix that is filled with ones.
## Create a matrix of shape (3, 3) filled with ones ## Here we have specified dtype = nm.int16 which asks NumPy to generate integers print(nm.ones((3, 3), dtype=nm.int16))
Output:
We have made use of the extra parameter in the form of dtype=nm.int16 in case you need to generate a matrix with ones. The nm.ones functions are used to create integers apart from considering the float as a default one. The extra parameter is now passed to nm.zeros.
Matrix examples and operations
Let me explain different Matrix operations and examples.
Additions
There are two types of additions, namely Scalar addition and Matrix additions in this case of the matrix, and let them see below.
import numpy as nm ## Generate two matrices matx_2d_1 = nm.array([nm.arange(1,4), nm.arange(4,7)]) matx_2d_2 = nm.array([nm.arange(7,10), nm.arange(10,13)])</pre> print("Matrix1: n ", matx_2d_1) print("Matrix2: n ", matx_2d_2) ## Add 1 to each element in matx_2d_1 and print it print("addition_scalar: n ", matx_2d_1 + 1)
## Add two matrices above elementwise print("Addition of two matrices of same size element wise: n ", matx_2d_1 + matx_2d_2)
Output
Matrix1:
Matrix2:
Addition_scalar:
Addition of two matrices of same size element-wise:
Subtraction
The process of subtraction matrix is identical to addition. You need to change the operation to subtraction from addition.
import numpy as nm ## Generate two matrices matx_2d_1 = nm.array([nm.arange(1,4), nm.arange(4,7)]) matx_2d_2 = nm.array([nm.arange(7,10), nm.arange(10,13)]) print("Matrix1: n ", matx_2d_1) print("Matrix2: n ", matx_2d_2) ## Subtract 1 from each element in matx_2d_1 and print it print("Scalar subtraction: n ", matx_2d_1 - 1) ## Subtract two matrices above elementwise print("Subtraction of two matrices of same size element wise: n ", matx_2d_1 - matx_2d_2)
Output:
The output of the above matrix will be
Matrix1:
Matrix2:
Scalar subtraction:
Subtraction of two matrices of same size element-wise:
Product:
Scalar products and dot products are the two types of product operation done on NumPy matrices.
Scalar product: a scalar value is calculated by multiplying all the matrix elements.
Dot product: it is defined as the product of two matrices and also matches the matrix multiplication rules.
Let’s check with an example.
import numpy as nm ## Generate two matrices of shape (1,2) and (2,1) so that we can find ## dot product matx_2d_1 = nm.array([nm.arange(1,4), nm.arange(4,7)]) matx_2d_2 = nm.array([nm.arange(1,3), nm.arange(3,5), nm.arange(5,7)]) ## Print shapes and matrices print("Matrix1: n ", matx_2d_1) print("Matrix1 shape: n", matx_2d_1.shape) print("Matrix2: n ", matx_2d_2) print("Matrix2 shape: n", matx_2d_2.shape) ## Multiply each element by 2 in matx_2d_1 and print it print("Scalar Product: n ", matx_2d_1 * 2) ## Finding product of two matrices with the help of dot product print("Dot Product: n ", nm.dot(matx_2d_1, matx_2d_2))
In the above code, we have used * operator, and this is used only for the scalar multiplications. We make use of the function nm.dot() in the case for the matrix multiplication, and this indicated it takes two NumPy 2D arrays as an argument.
Output
Matrix1: n
Matrix1 shape: n (2, 3)
Matrix2: n
Matrix2 shape: n (3, 2)
Scalar Product: n
Dot Product: n
Division:
The division can be done with the help of the’/’, which is the division operator.
import numpy as nm ## Generate a matrix of shape (2,4) matx_2d = nm.array([nm.arange(1,4), nm.arange(4,7)]) ## Print the matrix print("Matrix: n ", matx_2d) ## Element wise division by scalar print("Scalar Division: n ", matx_2d / 2)
Output:
Matrix: n
Scalar Division: n
Exponent:
The exponent can be performed by the operator ‘**’
Let’s check out the code for Exponent.
import numpy as nm ## Generate a matrix of shape (2,4) matx_2d = nm.array([nm.arange(1,4), nm.arange(4,7)]) ## Print the matrix print("Matrix: n ", matx_2d) ## Find exponent element-wise print("exponent: n ", matx_2d ** 2)
Output:
Matrix: n
exponent: n
I hope the above tutorial helped you to know about implementing the matrices with NumPy. Any doubts? Contact us through the comment section below.