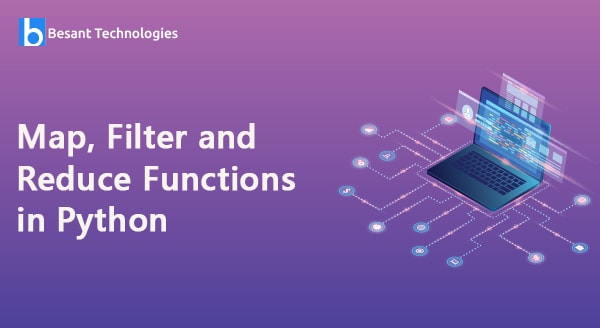
Map,Filter and Reduce Functions in Python
Introduction
Python is having a lot of inbuilt functions in predefined libraries. That functions will normally be classified into some categories like user-defined functions, Predefined Functions, and Anonymous Functions. Basically these functions are used to ease the programs as well as create a standard code environment also.
In This tutorial we going to discuss some predefined functions of python as map(), reduce() and filter() functions. Normally these functions will consider as Higher-Order Functions of python. Higher-Order functions Mean a function that will act as a parameter of another function. These functions are also used for enabling the functional programming aspects in python. In functional programming, arguments of the function will decide the output.
Lambda function:
Before we start about Higher-order functions we need some knowledge about lambda functions. Because lambda is used to achieve the above functions implementation.
Lambda is an operator or function used to create an anonymous function (a function without a name). It is also called throw-away functions (i.e needed when they are created.)
General Format:
- lambda arquments_list: expression
- lambda: Keyword for declare lambda functions.
- arquments_list: pass the arguments for the expressions. Arguments are separated by a comma.
- Expression: Operations to be the process of output we wanted.
Example:
x = lambda a: a*a print(“Square is:”,x(5))
In the above example finding the square of the given number using lambda function. Here we are not using any function name just use the lambda keyword and pass the argument value with expression. But here we can use x as the name for lambda.
map() Function
map() is a function is takes the sequence of iterable values as a parameter to another function and created the results as list. Optionally we can also provide more iterables as a parameter to map and the result will be a list of tuples. And also it returns the iterator it will cast to list.
Syntax:
map(function,Iterable,..)
Here, the function is an expression to be processed for iterables whereas iterable is the sequence of values like list, tuples and etc.,
Example:
n=[8,3,4,6,9,12,14,9,5,16] output=list(map(lambda x:x%5,n)) print(output)
Output:
[3, 3, 4, 1, 4, 2, 4, 4, 0, 1]
Here this example we take lambda function to process the map(). Take the x as an argument a Set the expression as x%5 and take the Iterable n. now the n will become x%5. The result of this program is all the values of iterables n will be converted into modulo 5 values and stored in another list called output.
Output
[3, 3, 4, 1, 4, 2, 4, 4, 0, 1]
In the above example we are using list() other than list we can use tuple() and set() function for corresponding to immutable as well as ordering of values. Now we will see another example for map() with a user-defined function.
Example:
def func_1(n): return n*5 nums=[1,2,3,4,5] x=set(map(func_1,nums)) print(x)
In this example, we using the user-defined function called func_1(parameter). It will return the value of n*5. This example map() will pass the iterable nums and call the func_1(n) and result in the values as n*5 to the set(). So that the order of the output will differ.
Output:
{25, 10, 20, 5, 15}
map() Example with tuple()
Example:
def func_1(n): return n*5 nums=[1,2,3,4,5] x=tuple(map(func_1,nums)) print(x)
filter() function
The filter() function returns the output values of the iterables based on the true condition. Because filter() function having working principles of condition. It will also reduce the length of the iterables.
Syntax:
filter(function,iterables)
Here, function is a expression to be process for iterables where as iterable is the sequence of values like list.
In filter() also we can use both userdefined as well as lambda() to process.
Example:
n=[4,5,6,7,3,6,8,2,4,5] out=list(filter(lambda x:x>=5,n)) print(out)
In this example, we have iterable as a list named n. n is pass as a parameter to the filter function with lambda expression of x>=5. If the values of n is matched the condition and became True means that values should be made the output as list again. False values not taken by the output list.
Output:
Out= [5, 6, 7, 6, 8, 5]
Using filter() with User Defined Function
Example:
def func_2(n): if n>=5: return n n=[4,5,6,7,3,6,8,2,4,5] out=list(filter(func_2,n)) print(out)
In this example out is the result of values n with the filter output. It returns the true when the values of n is matched with n>=5.
Output:
[5, 6, 7, 6, 8, 5]
reduce():
The reduce() function name itself is the description it’s going to reduce the iterable as one value. It also returns the output as cumulative sum of results. It must have two parameters for the function. If supposed to use initializer for reduce() initializer should be the first arqument. It requires module importing when using in python 3 version.
from functools import reduce
Syntax:
reduce(function,iterables)
Here the function is the expression to be processed for iterables. Iterables is the collection of values like a list.
Example:
from functools import reduce
n=[1,2,3,4,5] x=reduce(lambda a,b:(a+b),n) print(x)
For this example, we can pass the list n values as a parameter and it returns the result of the values of addition to output x as single value. Here lambda will take two parameters a and b because in reducing we must pass two parameters for function and it will return a single value as a result.
Output:
X=10
Using Higher Order functions with each other (map(),reduce() and filter() ):
Its also possible we can use the functions like map(),filter() and reduce() along used in each other. (i.e pass the filter() function as a parameter to map() an vice versa.) for this internal function will process first and the outer function.
Using filter() in map() function:
if you are trying to use filter() in map() function. First, the filter() will reduce the iterable based on condition and then map() will match the result.
Example:
n=[1,2,3,4,5] x=list(map(lambda a:a%2,filter(lambda a:a>=3,n))) print(x)
In this example first the filter() function filters the iterables values n as a>=3. Then the map() will match the filtered result into a%2 and stored in x.
Output:
[1, 0, 1]
Using map() in filter() function:
If you are trying to implement a map() in filter(). The first map() will be matched and convert the values into matched values and the filter() will reduce the iterable based on true condition.
Example:
n=[5,6,3,4,7,8,2,9] x=list(filter(lambda a:a>=3,map(lambda a:a%5,n))) print(x)
In this example, the first map() function will convert the values of iterable n as a%5. then the filter() function will match the values based on the true condition as a>=3.
Output:
[3, 4, 3, 4]
Using map() and filter() in reduce() function:
Here the result will be based on the condition supplied by the reduce() function.
Example:
from functools import reduce
n=[5,6,3,4,7,8,2,9] x=reduce(lambda a,b:a+b,map(lambda a:a%5,filter(lambda a:a>=5,n))) print(x)
In this example, all the processes of the map() and filter() will working based on reducing () function.
Output:
10