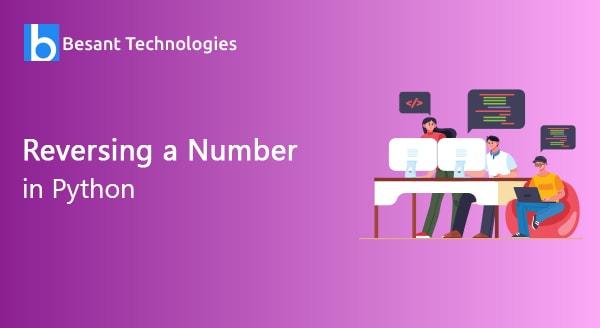
Reversing a Number in Python
Reversing a Number in Python
Python is a general-purpose programming language with the process of interpreted, high-level programming language. It also supports OOPS concepts. It mainly used to generate standard software applications. Understanding the concepts of python is helps us to create different types of Applications using python. For that, we use some fundamental programs to do it. That we also called implement the logic process in programs like finding prime no, even or odd no’s and etc..
How to reverse a number?
For those kinds of logic programs in this tutorial, we implement a logic called reversing a number. It is simple to implement. Just take a number as input and reverse the digits of the number. It will be done using some looping concepts. Take the input number in variable and check the condition repeatedly until the number is get reversed and stores the value in another variable.
In this reversing a number in python we have different formats to do. The following are the types of formats to reverse a number.
- Using a loop process.
- Using the recursive function.
Using a loop process
For this process probably we use the while loop to do this logic. Because while loop is considered the condition controlled loop in python. So that the number of iteration will be based on condition in this logic.
Example:
# Get the number from user using input() num = int(input("Enter The number for Reverse: ")) # Initiate temp value to 0 rev_num = 0 # Check using while loop while(num>0): #Logic remainder = num % 10 rev_num = (rev_num * 10) + remainder num = num//10 # Display the result print("The reverse number is :",rev_num)
Output:
Enter The number for Reverse: 654321 The reverse number is : 123456
For this logic, we need important operators %(modulo) and // (floor division). Because these two operators help us to reduce the digits as well as get the individual number to implement the logic.
Let discuss how this output will be processed in the while loop.
First, we need the variable to store the input value. Here we take num as an input variable and rev_num as output variable.
Iteration 1:
Before iteration starts to ensure the num value is not 0 or other types of value. Now starts the iteration. While will check the condition until num>0.
Before Iteration1 : num = 654321 , rev_num =0 Now we implement the logic. remainder = num % 10 (why 10 means it is a decimal number, modulo value of num is stored in the remainder) remainder = 654321 % 10 (remainder = 1) rev_num = (rev_num * 10) + remainder ( the logic for reversing digit position is multiply with 10) rev_num = (rev_num * 10) + 1 (rev_num = 1) num = num // 10 num = 654321 // 10 (num = 65432)
Iteration 2:
After the first iteration value of num will be changed to 65432. And rev_num is 1. Before Iteration2 : num = 65432 , rev_num =1 Now we implement the logic. remainder = num % 10 (why 10 means it is a decimal number, modulo value of num is stored in the remainder) remainder = 65432 % 10 (remainder = 2) rev_num = (rev_num * 10) + remainder ( the logic for reversing digit position is multiply with 10) rev_num = (rev_num * 10) + 2 (rev_num = 12) num = num // 10 num = 65432 // 10 (num = 6543)
Iteration 3:
After the second iteration value of num will be changed to 6543. And rev_num is 12.
Before Iteration 3 : num = 6543 , rev_num =12 Now we implement the logic. remainder = num % 10 (why 10 means it is a decimal number, modulo value of num is stored in the remainder) remainder = 6543 % 10 (remainder = 3) rev_num = (rev_num * 10) + remainder ( the logic for reversing digit position is multiply with 10) rev_num = (rev_num * 10) + 3 (rev_num = 123) num = num // 10 num = 6543 // 10 (num = 654)
Iteration 4:
After the third iteration value of num will be changed to 654. And rev_num is 123.
Before Iteration 4: num = 654 , rev_num =123 Now we implement the logic. remainder = num % 10 (why 10 means it is a decimal number, modulo value of num is stored in the remainder) remainder = 654 % 10 (remainder = 4) rev_num = (rev_num * 10) + remainder ( the logic for reversing digit position is multiply with 10) rev_num = (rev_num * 10) + 4 (rev_num = 1234) num = num // 10 num = 654 // 10 (num = 65)
Iteration 5:
After the fourth iteration value of num will be changed to 65. And rev_num is 1234.
Before Iteration 5: num = 65 , rev_num =1234 Now we implement the logic. remainder = num % 10 (why 10 means it is a decimal number, modulo value of num is stored in the remainder) remainder = 65 % 10 (remainder = 5) rev_num = (rev_num * 10) + remainder ( the logic for reversing digit position is multiply with 10) rev_num = (rev_num * 10) + 5 (rev_num = 12345) num = num // 10 num = 65 // 10 (num = 6)
Iteration 6:
After the fifth iteration value of num will be changed to 6. And rev_num is 12345.
Before Iteration 6: num = 6 , rev_num =12345 Now we implement the logic. remainder = num % 10 (why 10 means it is a decimal number, modulo value of num is stored in the remainder) remainder = 6 % 10 (remainder = 6) rev_num = (rev_num * 10) + remainder ( the logic for reversing digit position is multiply with 10) rev_num = (rev_num * 10) + 6 (rev_num = 123456) num = num // 10 num = 6 // 10 (num = 0)
The next iteration will not happen because num will be zero for our logic number should be greater than zero so that number will be ended. Now print the result stored in rev_num as 123456.
Reversing Number with Recursive
num = int(input("Enter any Number to reverse: ")) result = 0 def Rev_Int(num): global result if(num > 0): Reminder = num %10 result = (result *10) + Reminder Rev_Int(num //10) return result result = Rev_Int(num) print("Reverse value of the given number is = %d" %result)
Output:
Enter any Number to reverse: 321 Reverse value of the given number is = 123