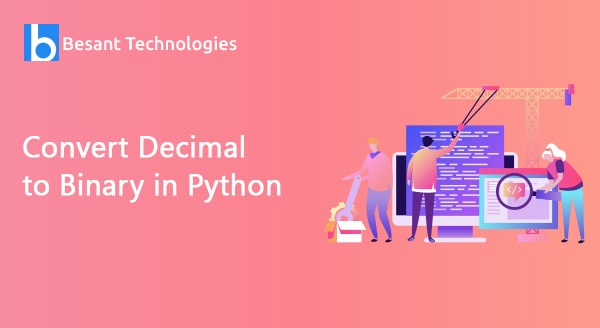
Convert Decimal to Binary in Python
Python is a general-purpose programming language with the process of interpreted, high-level programming language. It also supports OOPS concepts. It mainly used to generate standard software applications. Understanding the concepts of python is helps us to create different types of Applications using python. For that, we use some fundamental programs to do it. That we also called implement the logic process in programs like finding prime no, even or odd no’s and etc..
In this tutorial, we study converting decimal to binary logic. Let see what decimal and binary values are. The number having the base 10 is called decimal and base 2 as binary. These numbers can able to convert one format into another.
Example:
From decimal to binary Decimal number: 8 (input) Binary format: 1000 (output) From binary to decimal Binary number: 1001 Decimal format: 9
Converting a Decimal number to Binary
Converting decimal to binary will happen to the following steps.
- Repeatedly do the function of n/2.
- Check the number until the n>1.
- Then do the n%1 for getting binary number.
Example:
Take the input decimal number as 9.
- 9/2. Get the quotient as 4 (n>1) and remainder as 1.
- 4/2. Get the quotient as 2 (n>1) and remainder as 0.
- 2/2. Get the quotient as 1 (not greater n>1). And remainder as 0.
- 1%2. Get the remainder as 1.
Now write the output from last to first step remainder values like 1001.
Input: 9 Output: 1001
Now let us implement this concept in the python program.
Example:
# Function to print binary number for the # input decimal using recursion def decimal_To_Binary(n): if(n > 1): # divide with integral result # (discard remainder) decimal_To_Binary(n//2) print(n%2, end=' ') # Driver code if __name__ == '__main__': decimal_To_Binary(8) print("") decimal_To_Binary(9) print("") decimal_To_Binary(10) print("")
Output:
1 0 0 0 1 0 0 1 1 0 1 0
In this example, we convert the decimal numbers into binary using the above following logic using divide the decimal number into 2. Up to n>1 after that using the n%2 to get the last value.
Learn Python from the Basic to Advanced Level with Hands-on Training, Placements, and more with
Python Training in Bangalore
Convert the decimal number into binary using bin function
In python it is also possible to convert the decimal number into binary using a function call bin (n). Just pass the decimal number and it converts the binary value.
Example:
#Function to convert Decimal number # to Binary number def decimal_To_Binary(n): return bin(n).replace("0b","") # Driver code if __name__ == '__main__': print(decimal_To_Binary(8)) print(decimal_To_Binary(9)) print(decimal_To_Binary(10))
Output:
1000 1001 1010
In this example, we implement the decimal to binary conversion using bin() function. In this example, we just pass the decimal number as the argument of bin(n) function. And it returns the binary format of the decimal number with an indication of ‘0b’ in prefix. So that we replace that value using “. Then we get an exact binary format.
Get Placement Oriented Python Training from Industry Experts with our Python Training in Chennai
Convert Binary to Decimal:
Let us see the vice versa process. i.e converting binary into a decimal number. Here we take binary values as input (0 or 1). And convert into an exact decimal number.
Conversion steps as follows.
Example:
1001
- Take the binary number and use the modulo operator to split the values using decimal 10. (1001%10 = 1)
- Multiply the remainder with power of 2 as 0. It will be increased based on iteration like 2^0,2^1,.. Until the last iteration. But take the values from the right.
- Add the values to result. Result= result + (2**0)
- Decrease the binary using //10. 1001 // 10.
- Repeat the process until the last digit i.e n>0.
Final Result is:
1001 = 1+(2**3)+0+(2**2)+0+(2**1)+1+(2**0) = 8 +0 +0+1 = 9
Example:
# Function calculates the decimal equivalent # to given binary number def binary_To_Decimal(b): b1 = b deci, i, n = 0, 0, 0 while(b != 0): dec = b % 10 deci = deci + dec * pow(2, i) b = b//10 i += 1 print(deci) # Driver code if __name__ == '__main__': binary_To_Decimal(111) binary_To_Decimal(1000) binary_To_Decimal(1001)
In this example, we implement the conversion of binary to decimal to use the above steps for conversion.
Output:
7 8 9
Convert binary to decimal using int() function:
It is also possible to convert binary to decimal using int() function. Here we pass the binary values as a string in the parameter then use the int function with the format of binary 2. Like int(n,2). Here n is the string value and 2 is the binary format value.
Example:
# Function to convert Binary number # to Decimal number def binary_To_Decimal(n): return int(n,2) # Driver code if __name__ == '__main__': print(binary_To_Decimal('0111')) print(binary_To_Decimal('1000')) print(binary_To_Decimal('1001'))
In this example, we implement binary to decimal conversion using int() function.
Learn Python Course to Boost your Carrer with our Python Online Training