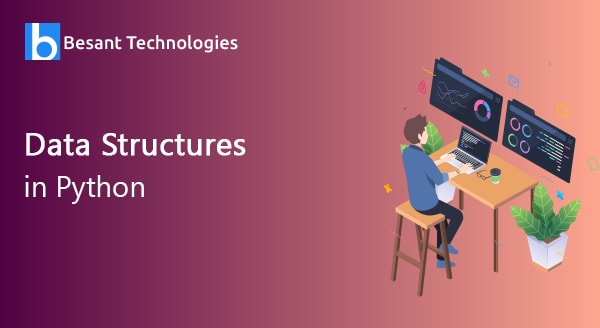
Data Structures in Python
Data Structures in python
Python can able to create different types of applications like web, desktop, Data Science, Artificial Intelligence and etc… for creating that kind of application mostly possible using data. Data is playing an important role that means data stored inefficiently as well as access in a timely. This process will be done using a technique called Data Structures.
What is Data Structures?
Data Structure is a process of manipulates the data. Manipulate is a process of organizing, managing and storing the data in different ways. It is also easier to get the data and modify the data. The data structure allows us to store the data, modify the data and also allows to compare the data to others. Also, it allows performing some operations based on data.
Types of Data Structures in python
Generally, all the programming language allows doing the programming for these data structures. In python also having some inbuilt operations to do that. Also in python, it divided the data structures into two types.
- Built-in Data Structures
- User-Defined Data Structures
Built-in Data Structures
Python having some implicit data structure concepts to access and store the data. The following are the implicit or Built-in Data structures in python.
- List
- Tuple
- Dict
- Set
These above four container data types are considered as built-in data structures in python. These data types having predefined methods to manipulate the data like storing, accessing and related operations.
List:
The list is a collection of elements with duplicates. It is also called ordered collection i.e what order you insertion the elements the same order it will return. It also follows the index-based storage so that the elements are arranged in order. It is also needed not to be homogeneous i.e storing similar types of values. But in a list, you can store different types of elements also. It is mutable also we can able to manipulate the elements like adding, removing and changing the values.
Creating a list:
Creating the list using square brackets as well as list constructor. List also be empty because it is mutable. If you can add the elements later.
Example:
my_l1=[] print(my_l1) my_l2=[1,2.5,’hello’] print(my_l2) my_l3=list([1,2,3,4,5]) print(my_l3)
Output:
[] [1,2.5,’hello’] [1,2,3,4,5]
Adding the Elements
You can add the elements in the list using the following methods.
- append() – This method used to the elements at the end of the list.
- insert() – It is also used to add the elements in the list. But not at the end of the list it adds the elements in the specified index.
- extend() – It is used to adds the list of elements to another list.
Example:
l=list() l.append (10) l.append(20) l.append (30) l.append(20) print(l) l.insert (3,40) print(l) l2=[50,60] l.extend (l2) print(l)
Output:
[10,20,30,20] [10, 20, 30, 40, 20] [10, 20, 30, 40, 20, 50, 60]
Deleting the Elements
Delete the elements in a list is done by using the following methods.
- remove() – It will remove the specified element in the list.
- pop() – It will remove the last element of the list straight away. If you pass index as a parameter then it removes the element in the index.
- clear() – It used to clear all the elements in the list.
- del – this keyword also used to remove the specified index element in the list.
Example:
l=[1,2,3,4,5] print(“List:”,l) del l[3] print(“after del:”,l) l.remove(3) print(“after remove”,l) l.pop() print(“after pop”,l) l.clear() print(“after clear”,l)
Output:
List:[1,2,3,4,5] After del : [1,2,3,5] After remove: [1,2,5] After pop:[1,2] After clear: []
Learn Python from the Basic to Advanced Level with Hands-on Training, Placements, and more with
Python Training in Bangalore
Accessing the Elements:
Elements are accessed in the list is based on the index value. Also, we use some slicing concepts to access values in the list.
Example:
l1=[1,2,3,4,5] print(l1[3]) # it will return 3 indexed element as 4. print(l1[:]) # it will return all the elements in a list print( l1[1:])# it will return the elements from index 1. print(l1[:3])# it will return the elements upto index 3. print(l1[-1:]) # it will return the last element. print(l1[:-1]) # it will return the elements upto -1 i.e last element.
Output:
4 [1, 2, 3, 4, 5] [2, 3, 4, 5] [1, 2, 3] [5] [1, 2, 3, 4]
Other Methods:
Apart from the above methods list using the following methods for process some operations.
- index() – It returns the index value of the specified element.
- count() – It returns the number of occurrences of the specified element.
- copy() – It returns all elements of a list to another list.
- reverse() – It reverses the element’s index in the list.
- sort() – It sort the elements of a list in ascending order.
- len() – It returns the number of elements in the list.
Example:
l=[1,3,2,5,4,5] print(l.index(3) print(l.count(5) l1=l.copy() print(l1) print(len(l1)) l.reverse() print(l) l.sort () print(l)
Output:
1 2 [1,3,2,5,4,5] 6 [5, 4, 5, 2, 3, 1] [1, 2, 3, 4, 5, 5]
Dictionary
Dictionary is a mutable collection to store the elements in a key-value pair. For example, a college has more than a number of students if suppose we identify the student based on their name but its difficult because names may be duplicate. So that kind of scenario we used register number to identify this kind of element stored using a dictionary. Because the dictionary stores the register number as key and name as value. Dictionary doesn’t allow duplicate keys based on that student register number also a unique one.
Creating a Dictionary
Dictionary normally creating using object. Also, it will create using curly braces {}. All the elements of the dictionary are key and value pairs. Like list dictionary also creates as empty.
Example:
m_dict={} print(m_dict) m_dict1={‘a’:”apple”,’b’:”banana”} print(m_dict1)
Output:
{] {‘a’:” apple”,’b’:” banana”}
Adding and Changing the Elements:
In dict always used the key to do the operations. Just like the index in a list. To adding the elements in the dictionary you may use a key-value pair. And also wants to override or change the value also based on key only.
strong>Example:
<m_dict1={‘a’:”ant”,’b’:”banana”} print(m_dict1) m_dict1[‘c’]=”cat” # add the element to the dictionary print(m_dict1) m_dict1[‘b’]=”bee” # changing the value of the element using key print(m_dict1)
Output:
{‘a’: ”ant”, ’b’ : ”banana”} {‘a’:”ant” , ‘b’ : “banana” , ‘c’: “cat”} {‘a’:”ant” , ‘b’ : “bee” , ‘c’: “cat”}
Deleting the elements:
Delete the elements in the collection also uses the following methods.
- pop() – It will remove the element in the specified key value.
- popitem() – It will remove the first set of key-value pairs in the dictionary. It also returns the elements in a tuple.
- clear() – it clears the all key-value pair of elements.
Example:
m_dict = {'a':"ant" , 'b' : "bee" , 'c': "cat"} print(m_dict) print(m_dict.pop('c')) print(m_dict.popitem()) m_dict.clear () print(m_dict())
Output:
{'b': 'bee', 'c': 'cat', 'a': 'ant'} 'cat' ('b', 'bee') {}
Accessing the Elements:
Access to the elements of the dictionary will be done using keys like an index. And also we use the method called get(). Just pass the key as a parameter to receive the element value.
Example:
m_dict = {'a':"ant" , 'b' : "bee" , 'c': "cat"} print(m_dict) print(m_dict['b']) print(m_dict.get('c'))
Output:
{'b': 'bee', 'c': 'cat', 'a': 'ant'} bee cat
Other Functions:
Apart from the above manipulation methods, we have some different methods in the dictionary as follows.
- Items() – It will return all the key-value pairs of elements in a list of tuples.
- keys() – It will return only the keys as a list.
- values() – It will return the values as a list.
Example:
m_dict = {'a':"ant" , 'b' : "bee" , 'c': "cat"} print(m_dict) print(m_dict.items()) print(m_dict.keys()) print(m_dict.values())
Output:
{'b': 'bee', 'c': 'cat', 'a': 'ant'} dict_items([('b', 'bee'), ('c', 'cat'), ('a', 'ant')]) dict_keys(['b', 'c', 'a']) dict_values(['bee', 'cat', 'ant'])
tuple:
A tuple is an immutable collection of elements. I.e once the elements are entered into the tuples cannot be changed. Otherwise, it just looks like a list in python.
Creating a tuple
Tuples are created using parenthesis or using a tuple constructor.
Example:
my_t=(1,2,3,4) print(my_t)
Output:
(1,2,3,4)
Get Placement Oriented Python Training from Industry Experts with our Python Training in Chennai
Accessing Elements:
Example:
my_t=(1,2,3,4) print(my_t) for i in my_t: print(i) print(my_t[2]) print(my_t[:])
Output:
(1,2,3,4) 1 2 3 4 3 (1,2,3,4)
Appending Elements:
Adding the elements to a tuple is done using the ‘+’ symbol. It just concatenates the tuples of values.
Example:
my_t=(1,2,3,4) my_t1=(7,8,9) my_t=my_t+my_t1 print(my_t)
Output:
(1,2,3,4,7,8,9)
Other Functions
Tuples are not allowing any manipulation methods because it is considered as an immutable collection of values. So it using only the following methods.
- count() – It returns the number of occurrences of a particular element.
- index() – It returns the position of the particular element.
Example:
my_t=(1,2,3,2) print(my_t) print(my_t.count(2)) print(my_t.index(3))
Output:
(1,2,3,4) 2 2
Sets
Sets in Python are a mutable collection of elements unordered. Also sets not allows duplicates. i.e if you insert the same element in more than one time even it will take only once. This set operations are more equal to the arithmetic sets in mathematics.
Creating a Sets
Sets are created using curly or flower braces in python. It doesn’t allow duplicates. i.e values are unique.
Example:
my_s={1,2,3,4,3,5,2} print(my_s)
Output:
{1,2,3,4,5}
Adding Elements
Add the elements in the sets using add() method.
Example:
my_s={1,2,4,2} my_s.add(3) print(my_s)
Output:
{1,2,3,4}
Other Operations in Sets
The different operation methods of the sets in follows.
- union() – It used to do the unit operations of the set. Just concatenate the two sets without duplicates.
- intersection() – It returns the common values of both sets.
- difference() – It returns the difference values in the passed set. And also deletes the data represent in both sets.
- symmetric_difference() – It returns same process of difference() method. But it will return the which data remaining in both sets.
Example:
my_s1={1,2,3,4} my_s2={3,4,5,6} print(my_s1.union(my_s2)) print(my_s1.intersection(my_s2)) print(my_s1.difference(my_s2)) print(my_s1.symmetric_difference(my_s2))
Output:
{1,2,3,4,5,6} {3,4} {1,2} {1,2,5,6}
User-Defined Data Structures
User-defined data structures are not predefined or built-in functions this kind of data structure is achieved using writing based on their algorithm logic. All languages follow the same. Here in python also we implement that data structures. Here the following are the user-defined data structures.
- Stack
- Queue
- Tree
- Linked list
- Graph
- HashMaps
Before starts with user-defined data structures, we need the difference between the arrays and the list. Arrays are used to store homogenous data into it also not a dynamic. Whereas the list in python is used to store heterogeneous data to store with dynamic.
Stack
A stack is the basic data structure concept that will be followed by virtual memory. It is also a linear data structure.It is followed to manipulate data in the order of LIFO Last In First Out process. All programming language memory managements will follow this data structure to store and retrieve the variables. Here the stack having some manipulation process like adding the element is a called pushing, removing the elements called poping and also access the elements on one side. That is called a TOP element. TOP will consider as a cursor to point the top element i.e current element of the stack. Once you pop the elements this cursor will move to one by one. Mostly stack will use in the recursive process and reverse the process.
Queue:
Like stack, Queue also a linear data structure follows the process of FIFO(First In First Out). Just opposite to stack. Here the first inserted element will be popped out first. Whereas in LIFO will pope out the last element inserted. The queue is created using an array of elements and operations are done in both ends of the queue like front-back or head-tail of process. Here we have two kinds of a queue that are en-queue and deque. Based on the operations adding and removing elements these two queues are used. The queue is mostly used in OS for scheduling the jobs and also used in network buffer management for traffic congestion and more.
Tree:
A tree will be the type of non-linear data structure. It stored the elements in a tree structure like parent and child node process. It also follows the root and nodes. The root will be the start element and nodes are the other data stored points. A tree having the levels indicates the depth of the information. The last node is also called leaves. A tree is used for efficiency in searching and more. It also used in website creation using Html to identify the enclosed tags.
Linked List:
The linked list also comes under the linear data structure type. It is stored the elements based on the nodes. Each node having the data and address for the next node. The data in the linked list is not stored inconsequently. This type of data structure is mostly used in music player applications and also image view applications. These having some different types also like doubly, circular.
Graph:
Graphs represent the stored data in collection with vertices (nodes ) and edges. These graphs are having the advantage to show the accuracy of data in real-world maps. These graphs are mostly used to finding the least path i.e cost-to-distance between the data points. Also called nodes. It mostly used in map-related applications like google maps, ola, uber for finding the shortest distance for better performance.
HashMaps:
HashMap is used to store the data in key-value pairs like a dictionary in python. These kinds of data structures are mostly used to store student details, phone books, grocery details storing and populating the data to list and much more.
Learn Python Course to Boost your Career with our Python Online Training