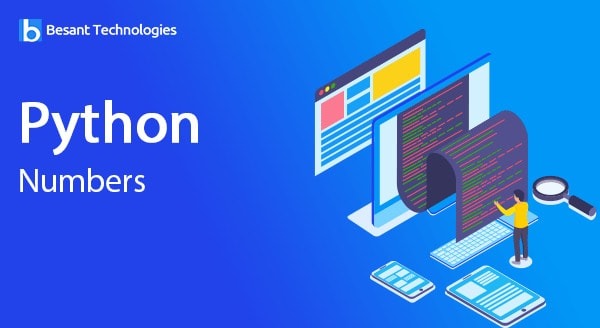
Python Numbers
Python Numbers
Basically, Python offers four kinds of numbers: integers, floats, complex numbers, and Booleans. Numbers in Python have two advantages over C or Java: Integers can be arbitrarily large, and the division of two integers results in a float. An integer constant can be -0, -11, +33, 123456- and has unlimited range, restricted only by the resources of your machine. A float can be a decimal point or in scientific notation: 3.14, -2E-8, 2.718281828. The precision of these values is governed by the underlying machine but is equal to double (64bit) types in C. Booleans are either True/False and behave identically to 1&0 except for their string representations.
Operations involving two integers produce an integer, except for division, which results in a float. If the “//” division symbol is used we get the result is an integer, with truncation. Operations involving a float always produce a float.
Here are a few examples:
>>5 + 2 - 3 * 2 1
floating-point result with normal division
>>> 7 / 2 3.5
also a floating-point result
>>> 7 / 2.0 3.5
integer result with truncation when divided using “//”
>>> 7 // 2 3
This would be too large to be an int in many languages
>>>3000000000 3000000000
>>>3000000000 * 3 9000000000 >>>3000000000 * 3.0 9000000000.0
Scientific notation gives back a float
>>>3.0e-8 3e-08
>>>300000 * 3000000 900000000000 >>>int(300.2) 300 >>>int(3e2) 300 >>>float(300) 300.0
These are explicit conversions between types 1. int truncates float values.
Built-in numeric functions:
Python provides below number related functions as part of its core:
abs, divmod, float, hex, int, max, min, oct, pow etc
Advanced numeric functions
More advanced numeric functions such as the trig and hyperbolic trig functions, as well as a few useful constants, aren’t built into Python but are provided in a standard module called math. So we can use below statement
math import *
The math module provides the following functions and constants:
acos, asin, atan, atan2, ceil, cos, cosh, e, exp, fabs, floor, fmod, frexp, hypot, ldexp, log, log10, mod, pi, pow, sin, sinh, sqrt, tan, tanh
Numeric computation
The core Python installation isn’t best suited to intensive numeric computation because of speed constraints. Even then, the powerful Python extension “NumPy” provides highly efficient implementations of many advanced numeric operations. The emphasis is on array operations, including multidimensional matrices and more advanced functions such as the Fast Fourier Transform. You should be able to find NumPy (or links to it) at www.scipy.org (http://www.scipy.org).
Click Here-> Python Interview Questions and Answers
Complex numbers
Complex numbers are created automatically whenever there is an expression of the form “nj” , with n having the same form as a Python integer or float. j is, of course, standard notation for the imaginary number equal to the square root of –1, for example:
>>> (3+2j) (3+2j)
Note that Python expresses the resulting complex number in parentheses as a way of indicating that what’s printed to the screen represents the value of a single object:
>>> 3 + 2j - (4+4j) (-1-2j) >>> (1+2j) * (3+4j) (-5+10j) >>> 1j * 1j (-1+0j)
Calculating j * j gives the answer of –1, but the result remains a Python complex number object. Complex numbers will never be converted automatically to equivalent real or integer objects. But can easily access their real and imaginary parts with real and imaginary:
>>> z = (3+5j) >>> z.real 3.0 >>> z.imag 5.0
Note that real and imaginary parts of a complex number are always returned as floating-point numbers.
Advanced complex-number functions
The functions in the math module don’t apply to complex numbers; the rationale is that most users want the square root of –1 to generate an error, not an answer! Instead, similar functions, which can operate on complex numbers, are provided in the cmath module:
acos, acosh, asin, asinh, atan, atanh, cos, cosh, e, exp, log, log10, pi, sin, sinh, sqrt, tan, tanh.
To make clear in the code that these functions are special-purpose complex-number functions and to avoid name conflicts with the more normal equivalents, it’s best to import the cmath module by saying
import cmath
and then to explicitly refer to the cmath package when using the function:
>>> import cmath >>> cmath.sqrt(-1) 1j Minimizing from <module> import *
This is a good example of why it’s best to minimize the use of the from <module> import * form of the import statement. If you used it to import first the math module and then the cmath module, the commonly named functions in cmath would override those of math. Certain modules are explicitly designed to use this form of import.
The important thing to keep in mind is that by importing the cmath module, you can do almost anything you can do with other numbers.
Click Here-> Get Python Training with Real-time Projects
Try this: Manipulating strings and numbers
In the Python shell, try to create some string and number variables (integers, floats, and complex numbers). Try multiplying a string by an integer, for example, or can you multiply it by a float or complex number? Also load the math module and try a few of the functions; then load the cmath module and do the same. What happens if you try to use one of those functions on an integer or float after loading the cmath module?
The None Value
In addition to standard types, Python has a special basic data type that defines a single special data object called None which is used to represent an empty value.
It is often useful in day-to-day Python programming as a placeholder to indicate a point in a data structure where meaningful data will eventually be found, even though such data hasn’t yet been calculated. We can easily test for the presence of None because there’s only one instance of None in the entire Python system (all references to None point to the same object), and None is equivalent only to itself.