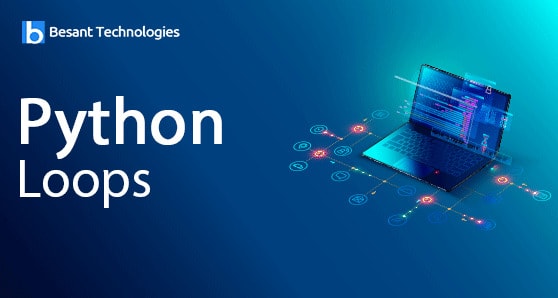
Python Loops
Python Loops
Loop statements which are used to be executed multiple times a repeated block of code and statements which are specified by the user
2 types of loops are listed below:
- While looping
- For looping
Python Iteration statement:
Python support three different looping statements.
While loop
While loop in python can be only used when the given conditions are true to execute a bock statement And when the condition is false .the control will come out of the loop.
While loop is used to executing multiple times of statement or codes repeatedly until the given condition is true once it gets a false loop will stop .which will be done by three parts initial value, condition, increment or decrement based this only while loop is working. the below parts are main.
- Initial Value.
- Condition
- Increment and Decrement value.
Based on while we can do the looping for multiple times and which can be loop the string,List, tuple,set and dict values also by using indexing we can retrieve the value of string,list, tuple,set and dict. While loop can even done through by below syntax.
Syntax:
while expression: statement(s) Increment or Decrement
In while loop, will check the expression, then if the condition expression becomes to be true, only then the block of statements to be executed if the condition expression becomes to be false.The while can we done by two way of program by Incremental form and decremental ordered.
The Increment can be done by the condition should be compare the smaller with bigger and Initial value is smaller than conditional value and The decrement can be done by the condition should be compare the bigger with smaller and Initial value is bigger than conditional value.
Example:
Step for Increment loop
a=1 while(a<=10): print a a+=1
Follow the steps below for Increment loop:
Step1:
We need to assign a value with start value with the smaller value rather than the conditional value so that loop will get started or else loop can’t be run through.
Step 2:
We need to set a condition based on start value as smaller once the condition gets pass the statements gets executed till the condition gets failed.
Step 3:
We need to set an increment value-based the needs of the function or statement the statement of loop is based on the increment value.
Program:
>>a=1 >>while(a<=10) print a a+=1
Output
1 2 3 4 5 6 7 8 9 10
Example:
Step for Decrement loop
a=10 while(a>=1):
print a a=a-1
Follow the steps below for decrement loop:
Step1:
We need to assign a value with start value with the bigger value rather than the conditional value so that loop will get started or else loop can’t be run through.
Step 2:
We need to set a condition based on start value as bigger value, once the condition gets pass the statements gets executed till the condition gets failed.
Step 3:
We need to set a decrement value-based on the needs of the function or statement the statement of loop is based on the decrement value.
Program:
>>while(a>=1) print a a-=1;
Output
10 9 8 7 6 5 4 3 2 1
Flow Chart – While Loop
Example of while loop:
x = 0 while (x < 5): print(x) x = x + 1
Output:
0 1 2 3 4
Learn Python from the Basic to Advanced Level with Hands-on Training, Placements, and more with
Python Training in Bangalore
While Loop with else in python:
Else part is executed if the condition part in while becoming false.
Syntax While Loop with else:
while (condition):
loop statements
else:
else statements
Example:
x = 1 while (x < 5): print('inside while loop value of x is ',x) x = x + 1 else: print('inside else value of x is ', x)
Output:
inside while loop value of x is 1 inside while loop value of x is 2 inside while loop value of x is 3 inside while loop value of x is 4 inside else value of x is 5
Example: Program to add natural
# numbers upto # sum = 1+2+3+...+n # To take input from the user, n = int (input("Enter n: ")) # initialize sum and counter sum = 0 i = 1 while i <= n: sum = sum + i i = i+1 # update counter # print the sum print("The sum is", sum)
For Loop in Python :
For loop in python is used to iterate of any sequence such as list or a string.
For loop:
For loop which is used to executing a block of statements or code several times until the given values are iterate able.
Syntax:
for variable in iterate:
statement(s)
Example:
Step for List of loop
x=[1,2,3,4,'a','b','c',0.1,0.2]
for i in x:
print i
Follow the Steps below for List value loop:
Step1:
We need to assign a value with list value to be iterating value rather than conditional value are not assigned.
Step 2:
We need to set a variable to passed in the statement and which are executed till the iterating values .
Step 3:
We no need to set a decrement /increment value based the needs of the function or statement the statement of loop is based on the list value.
Program
>>x=[1,2,3,4,’a’,’b’,’c’,0.1,0.2]
Output
1 2 3 4 a b c 0.1 0.2
Steps for String Value
x='Besant Technology'
for i in x:
print i
Follow the steps below for String value loop:
Step1:
We need to assign a value with String value to be iterating value rather than conditional value are not assigned.
Step 2:
We need to set a variable to passed in the statement and which are executed till the iterating values .
Step 3:
We no need to set a decrement /increment value based the needs of the function or statement the statement of loop is based on the String value.
Program:
>>x='Besant Technology' >>>for i in x print i
Output
B e s a n t T e c h n o l o g y
For loop using range () function:
Range () function is to generate a sequence of numbers from the start value to stop value based on step.
Syntax:
for variable in range(start,stop,step): statement(s)
Get Placement Oriented Python Training from Industry Experts with our Python Training in Chennai
Positive Range and Negative Range
Positive Range Example:
Step for For loop incremental
for i in range(1,10,1): print i
Follow the steps below for List value loop:
Step1:
We need to assign a value with range of value to be iterating value rather than conditional value are not assigned.
Step 2:
We need to set a variable to passed in the statement and which are executed till the iterating values .
Step 3:
We no need to set a decrement /increment value-based the needs of the function or statement the statement of loop is based on the range value.
Step 4:
We need to pass a value by assign a new variable
for i in range (1,10,1) print i
Output
1 2 3 4 5 6 7 8 9
Negative Range Example:
Step for For loop decrement
for i in range(10,0,-1): print i
Follow the steps below for List value loop:
Step1:
We need to assign a value with range of value to be iterating value rather than conditional value are not assigned.
Step 2:
We need to set a variable to pass in the statement and which are executed till the iterating values.
Step 3:
We no need to set a decrement /increment value-based the needs of the function or statement the statement of loop is based on the range value.
Negative Range
for i in range(10,0,-1) print i
Output
10 9 8 7 6 5 4 3 2 1
Positive Range access list value Example:
Step for For loop increment in the list value
x=’Beasnt Technology’ for i in range(0,len(x),1): print x[i]
Step1:
We need to assign a value with indexing of positive value to be iterating value rather than conditional value are not assigned.
Step 2:
We need to set a variable to pass in the statement and which are executed until the iterating values.
Step 3:
We no need to set a decrement /increment value-based the needs of the function or statement the statement of loop is based on the range value.
Program
>>x='Besant Technology' >>for i in range (0,len(x),1)
print x[i]
Output:
B e s a n t T e c h n o l o g y
Negative Range access list value Example:
Step for For loop decrement in the list value
x=’Beasnt Technology’ for i in range(len(x)-1,-1,-1):
print x[i]
Step1:
We need to assign a value with indexing of Negative value to be iterating value rather than conditional value are not assigned.
Step 2:
We need to set a variable to pass in the statement and which are executed until the iterating values.
Step 3:
We no need to set a decrement /increment value-based the needs of the function or statement the statement of the loop is based on the range value.
Program
>>x='Besant Technology' for i in range(len(x)-1,-1,-1) print x[i];
Output:
y g o l o n h c e T t n a s e B
For Loop syntax:
for val in sequence:
statements;
Flow Chart – For Loop
Example:
for i in range(1,5): print(i)
output :
1 2 3 4
Range function in python
It is built in function user to iterate over the sequence of number.
Syntax of range() function :
range(start, stop[, step])
The range() Function Parameters
- start Starting a number of the sequence.
- stop: Generate numbers up to, but not including this number.
- step(Optional): Determines the increment between each number in the sequence.
Example 1 : range() function
for i in range(5): print(i)
Output:-
0 1 2 3 4
Example 2 : range() function
for i in range(2,9): print(i) Run Code
Output:-
2 3 4 5 6 7 8
Learn Python Course to Boost your Career with our Python Online Training
Nested For Loops In Python
When one Loop defined within another Loop is called Nested Loops.
Syntax of Nested For Loops
for val in sequence:
for val in sequence:
statements
statements
Example 1: Nested For Loops (Pattern Programs)
for i in range(1,6): for j in range(0,i): print(i, end=" ") print('')
Output:-
1 2 2 3 3 3 4 4 4 4 5 5 5 5 5
Example 2: Nested For Loops (Pattern Programs)
for i in range(1,6): for j in range(5,i-1,-1): print(i, end=" ") print('')
Output:-
1 1 1 1 1 2 2 2 2 3 3 3 4 4 5