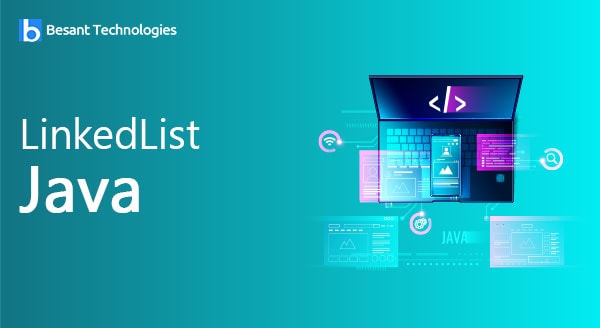
LinkedList Java
LinkedList Java
- Java LinkedList class uses doubly linked list to store the elements.
- It provides a linked-list data structure.
- It inherits AbstractList class and implements List and Deque.
- LinkedList is better for manipulating elements whereas and ArrayList is best fit for storing and retrieving the data.
Linked List Types
- Non generic form of LinkedList
- Generic form of LinkedList
Non generic form of LinkedList
Syntax:
LinkedList object = new LinkedList();
Generic form of LinkedList
Syntax:
LinkedList<DataType> object = new LinkedList<DataType>();
Example:
package Mypkg; import java.util.Iterator; import java.util.LinkedList; public class ListEx { /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub LinkedList<String> li=new LinkedList<String>();// generic collection - FIFO- FCFS- First Come First Serve li.add("Besant"); li.add("Technologies"); li.add("HSR Layout"); li.add("Sector-1"); li.add("Bangalore"); li.addFirst("India"); System.out.println("***********************************"); System.out.println("List Display" + li.toString()); System.out.println("**************************************"); li.removeFirst(); System.out.println("***********************************"); System.out.println("After Removal of First element" + li.toString()); System.out.println("**************************************"); li.remove(); System.out.println("***********************************"); System.out.println("After removal of an element" + li.toString()); System.out.println("**************************************"); li.addFirst("ttttt"); System.out.println("***********************************"); System.out.println("Adding element at first position" +li.toString()); System.out.println("**************************************"); System.out.println("Get elements of 2nd position " +li.get(2)); System.out.println("****Linked List items (Foreach Display)****************"); for(String d: li) { System.out.println(d); } System.out.println("********************************************************"); System.out.println("Linked List (Itertor Display)"); Iterator<String> iter=li.iterator(); while(iter.hasNext()) { System.out.println(iter.next()); } System.out.println("********************************************************"); } }
Output:
***********************************
List Display[India, Besant, Technologies, HSR Layout, Sector-1, Bangalore]
**************************************
***********************************
After Removal of First element[Besant, Technologies, HSR Layout, Sector-1, Bangalore]
**************************************
***********************************
After removal of an element[Technologies, HSR Layout, Sector-1, Bangalore]
**************************************
***********************************
Adding element at first position[ttttt, Technologies, HSR Layout, Sector-1, Bangalore]
**************************************
Get elements of 2nd position HSR Layout
****Linked List items (Foreach Display)****************
ttttt
Technologies
HSR Layout
Sector-1
Bangalore
********************************************************
Linked List (Itertor Display)
ttttt
Technologies
HSR Layout
Sector-1
Bangalore
********************************************************
Click Here-> Get Prepared for Java Interviews
Methods of LinkedList
Method Name | Description |
boolean add(E e) | Appends the element ‘e’ to the end of the list. |
void add(int i, E e) | Inserts the element ‘e’ at the specified index ‘i’ in the list. |
void addFirst(E e) | Inserts the element ‘e’ in the start of the list |
void addLast(E e) | Inserts the element ‘e’ in the end of the list |
void clear() | Removes all the elements from the list. |
boolean contains(Object o) | Returns true if the list contains the object specified in the argument. |
E get(int index) | Returns the element at the specified index in the list. |
boolean isEmpty() | Returns true if the list has no elements |
E remove(int index) | Removes the element at the specified index in the list. |
E removeFirst() | Removes and returns the first element in the list. |
boolean removeFirstOccurence(Object o) | Removes the first occurrence of the element in the list. |
E removeLast() | Removes and returns the last element in the list. |
boolean removeLastOccurence(Object o) | Removes the last occurrence of the element in the list (traversing from head to tail) |
E set(int index, E element) | Replaces the element at the specified index in the list with the element given. |
int size() | Returns the number of elements in the list. |
peek() | Retrieves the element in the head, but does not remove. |
E peekFirst() | Retrieves the first element of the list, but does not remove or returns null if this list is empty. |
E peekLast() | Retrieves the last element of the list, but does not remove or returns null if this list is empty. |
E poll() | Retrieves and removes the element in the head. |
E pollFirst() | Retrieves and removes the first element of the list. |
E pollLast() | Retrieves and removes the last element of the list. |
E pop() | Pops an element from the list, considering the list as stack. |
void push(E e) | Inserts the element e to the list, considering the list as stack. |
boolean addAll(Collection<? extends E> c) | Appends all of the elements in the specified collection to the end of the list. |
boolean removeAll(Collection<?> c) | Removes all the elements that are contained in the specified collection from the LinkedList. |
Click Here-> Get Java Training with Real-time Projects