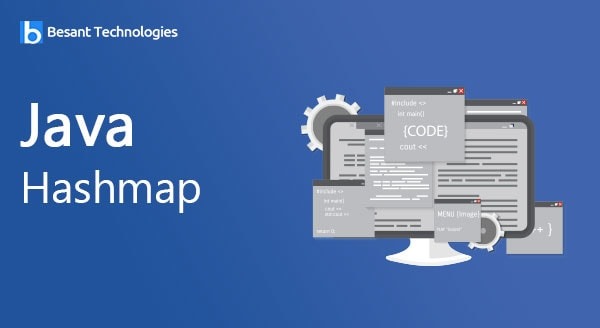
Java Hashmap
Java Hashmap
- A map contains values on the basis of key i.e. key and value pair.
- Each key and value pair is known as an entry. Map contains only unique keys.
- Map is useful if you have to search, update or delete elements on the basis of key.
Syntax of HashMap with Generic
Map<Integer,String> map=new HashMap<Integer,String>();
Example:
package Mypkg; import java.util.HashMap; import java.util.Hashtable; import java.util.Iterator; import java.util.Map; import java.util.Set; public class MAPex { /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub HashMap map=new HashMap(); //Adding elements to map map.put(1,"Neo"); map.put(3,"Rahul"); map.put(28,"Jai"); map.put(31,"Amit"); System.out.println("***********************"); System.out.println("Map Representation :"); System.out.println(map.toString()); System.out.println("***********************"); System.out.println( "Value against Key- 28->" + map.get(28)); //Traversing Map Set set=map.entrySet();//Converting to Set so that we can traverse Iterator itr=set.iterator(); System.out.println("Map elements are"); while(itr.hasNext()){ //Converting to Map.Entry so that we can get key and value separately Map.Entry entry=(Map.Entry)itr.next(); System.out.println(entry.getKey()+" -> "+entry.getValue()); } } }
Output:
***********************
Map Representation :
{1=Neo, 3=Rahul, 28=Jai, 31=Amit}
***********************
Value against Key- 28->Jai
Map elements are
1 -> Neo
3 -> Rahul
28 -> Jai
31 -> Amit
Click Here-> Get Prepared for Java Interviews
Method Name | Description |
void clear() | It is used to remove all elements from map. |
boolean isEmpty() | It is used to return true if this map contains no key-value mappings. |
Object clone() | It is used to return a shallow copy of this HashMap instance: the keys and values themselves are not cloned. |
Set entrySet() | It is used to return a collection view of the mappings contained in this map. |
Set keySet() | It is used to return a set view of the keys contained in this map. |
V put(Object key, Object value) | It is used to insert an entry in the map. |
void putAll(Map map) | It is used to insert the specified map in the map. |
V putIfAbsent(K key, V value) | It inserts the specified value with the specified key in the map only if it is not already specified. |
V remove(Object key) | It is used to delete an entry for the specified key. |
boolean remove(Object key, Object value) | It removes the specified values with the associated specified keys from the map. |
V compute(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) | It is used to compute a mapping for the specified key and its current mapped value (or null if there is no current mapping). |
V computeIfAbsent(K key, Function<? super K,? extends V> mappingFunction) | It is used to compute its value using the given mapping function, if the specified key is not already associated with a value (or is mapped to null), and enters it into this map unless null. |
V computeIfPresent(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) | It is used to compute a new mapping given the key and its current mapped value if the value for the specified key is present and non-null. |
boolean containsValue(Object value) | This method returns true if some value equal to the value exists within the map, else return false. |
boolean containsKey(Object key) | This method returns true if some key equal to the key exists within the map, else return false. |
boolean equals(Object o) | It is used to compare the specified Object with the Map. |
void forEach(BiConsumer<? super K,? super V> action) | It performs the given action for each entry in the map until all entries have been processed or the action throws an exception. |
V get(Object key) | This method returns the object that contains the value associated with the key. |
V getOrDefault(Object key, V defaultValue) | It returns the value to which the specified key is mapped, or defaultValue if the map contains no mapping for the key. |
boolean isEmpty() | This method returns true if the map is empty; returns false if it contains at least one key. |
V merge(K key, V value, BiFunction<? super V,? super V,? extends V> remappingFunction) | If the specified key is not already associated with a value or is associated with null, associates it with the given non-null value. |
V replace(K key, V value) | It replaces the specified value for a specified key. |
boolean replace(K key, V oldValue, V newValue) | It replaces the old value with the new value for a specified key. |
void replaceAll(BiFunction<? super K,? super V,? extends V> function) | It replaces each entry’s value with the result of invoking the given function on that entry until all entries have been processed or the function throws an exception. |
Collection values() | It returns a collection view of the values contained in the map. |
int size() | This method returns the number of entries in the map. |
Hash Table Vs HashMap
HashMap | Hashtable |
non synchronized. It is not-thread safe and can’t be shared between many threads without proper synchronization code. | synchronized. It is thread-safe and can be shared with many threads. |
allows one null key and multiple null values. | doesn’t allow any null key or value. |
faster. | Slower. |
HashMap is traversed by Iterator. | Hashtable is traversed by Enumerator and Iterator. |
Iterator in HashMap is fail-fast. | Enumerator in Hashtable is not fail-fast. |
HashMap inherits AbstractMap class. | Hashtable inherits Dictionary class. |
Click Here-> Get Java Training with Real-time Projects